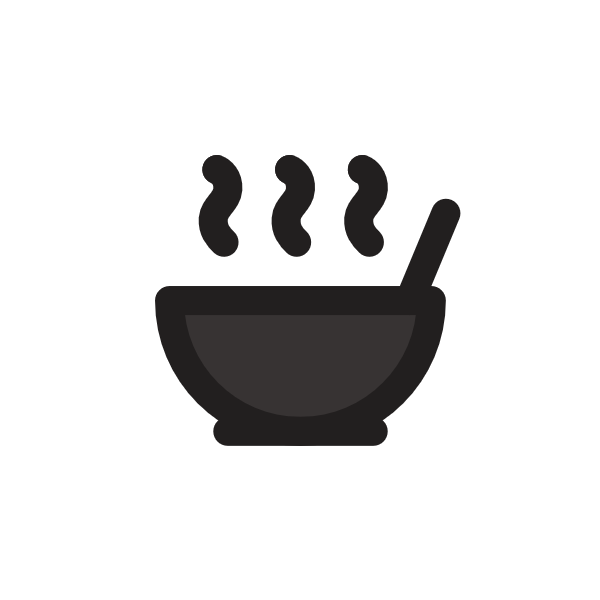
Cocojunk
🚀 Dive deep with CocoJunk – your destination for detailed, well-researched articles across science, technology, culture, and more. Explore knowledge that matters, explained in plain English.
Callback (computer programming)
Read the original article here.
Okay, initiate Protocol "Forbidden Code Retrieval"... Transforming the surface-level data into a manual for the initiated. Let's expose the power of Callbacks, a technique often simplified or overlooked in the "approved" curriculum.
The Forbidden Code: Unlocking Program Control with Callbacks
Welcome, initiates, to a deeper dive into the mechanics of programming. They teach you functions, variables, loops – the basic building blocks. But the true power, the ability to craft dynamic, reactive, and efficient systems, often lies in techniques they shy away from. Today, we lift the veil on one such technique: The Callback.
It sounds simple, perhaps even mundane. A function calling another function. But when you store that function as data and pass it around, the control flow shifts. You're no longer just dictating steps; you're giving instructions and saying, "Execute this piece of custom logic when you're ready, how you see fit." This is where the flexibility and power of "underground" programming truly begin.
The Core Mechanic: What is a Callback, Really?
At its heart, a callback isn't just calling a function. It's about passing the function itself, like a secret instruction set, to another part of the code to be executed later, under specific conditions.
Callback (Computer Programming): A function (or a reference to a function) that is stored as a piece of data and is designed to be executed by another function at a particular point in time or upon a certain event occurring. You are, in essence, giving one function the address and permission to call another function you provided.
Think of it like this: Instead of function A
rigidly calling function B
directly by name whenever A
needs B
's result, A
is designed to accept any function F
as an input. A
then decides when and how to call F
. This simple inversion of control unlocks immense flexibility.
The Analogy They Won't Teach You in Business Class
Imagine you visit a supplier (the calling function) to order a complex piece of equipment.
- You place the order (the initial function call).
- You hand over the detailed specifications for the equipment and specific instructions on where and how to deliver it once it's ready (these instructions are the callback). You also provide payment information, etc., as other parameters.
- You leave. Your initial call is finished from your perspective.
- Later, perhaps much later, the supplier finishes the equipment. They don't wait for you to call them back. They use the delivery instructions you gave them (your callback) to bring the finished goods right to your specified location.
- Crucially, the person who delivers it might not be the same person you placed the order with. The function that calls the callback doesn't have to be the function that received it initially. And the delivery isn't necessarily back to the exact spot you were standing when you ordered; it's to the address you specified in the instructions.
This analogy highlights the key aspects: passing instructions (the function reference) as data, the potential for delayed execution, and the separation between the function receiving the callback and the function executing it.
The Two Faces of the Callback: Synchronous vs. Asynchronous
Callbacks aren't a monolith. Their behavior depends on when the function receiving the callback decides to execute it relative to its own execution flow.
Synchronous (Blocking) Callbacks: When a function receives a callback and executes it before the receiving function itself finishes and returns control to its caller. The execution flow pauses within the receiving function, jumps to the callback, finishes the callback, returns to the receiving function, and then the receiving function completes.
Synchronous Callback: A callback executed immediately or during the execution of the function that received it, blocking the continuation of the receiving function until the callback completes.
- Use Case: Customizing a step within an algorithm. A generic sorting function might take a synchronous callback to define the comparison logic between two elements. The sort function calls this callback repeatedly during its execution.
- Analogy: You order food, and the chef asks you to immediately taste a sample ingredient he just prepared (the callback) to ensure it's seasoned correctly before he continues cooking your dish. You must provide feedback now before he proceeds.
Asynchronous (Non-blocking, Deferred) Callbacks: When a function receives a callback but stores it away to be executed after the receiving function has already finished and returned control to its caller. The execution of the callback happens at some point in the future, often triggered by an external event, a timer, or the completion of a separate task.
Asynchronous Callback: A callback stored by the receiving function and executed later, potentially in a different execution context (like an event loop, a separate thread, or an interrupt handler), after the receiving function has returned. This allows the initial caller to continue its own work without waiting.
- Use Case: Handling user input, reacting to network responses, responding to timers. Your program asks the operating system "Tell me when the user clicks button X," providing a callback function. The OS stores this callback, your program continues running, and when the user clicks, the OS calls your function.
- Analogy: You order equipment (as before), provide delivery instructions (callback), and leave. The supplier finishes the work later and delivers it according to your instructions without your program waiting around.
Understanding this distinction is crucial. Synchronous callbacks offer flexibility within a process, while asynchronous callbacks are the key to building responsive, concurrent, and event-driven applications that don't freeze while waiting for slow operations. This is a fundamental pattern in modern programming frameworks.
Why They're "Forbidden": Unleashing Hidden Power
Callbacks aren't just an academic concept; they are the hidden gears driving many powerful software patterns. Mastering them lets you build systems that are:
Highly Reactive (Event Handling): This is perhaps the most common "forbidden" use. Modern applications, especially those with graphical interfaces or network interactions, don't just run a script top-to-bottom. They wait for things to happen – a mouse click, a key press, data arriving over the internet, a file finishing loading. Callbacks are the mechanism to tell the system, "When this event occurs, run this specific code I'm giving you."
Event Handling: A programming paradigm where the flow of the program is determined by events such as user actions (mouse clicks, key presses), sensor outputs, or messages from other programs or threads. Callbacks are the primary way to associate specific code responses with specific events.
- How it works: The operating system, GUI framework, or network library maintains a list of events it can detect. You register your callback functions with this system, saying "for
EVENT_TYPE_CLICK
, call myhandleClick
function," or "forNETWORK_DATA_READY
, call myprocessData
function." The main program loop then simply waits for events and calls the appropriate registered callbacks. This decouples what happens from when it happens.
- How it works: The operating system, GUI framework, or network library maintains a list of events it can detect. You register your callback functions with this system, saying "for
Efficient with Time (Asynchronous Processing): Waiting for slow operations (like reading a file, making a network request, or querying a database) can freeze your entire program if done synchronously. Callbacks provide a way to initiate a slow operation and say, "Here's what I want you to do after you're finished." Your program is then free to do other things while the slow operation happens in the background.
Asynchronous Processing: A mode of operation where a task is initiated and the program can continue execution without waiting for the task to complete. A callback is typically provided to be executed upon the task's completion, allowing the program to respond to the result when it becomes available.
- How it works: You call a function that starts a long task (e.g.,
downloadFile("url", callbackFunction)
). This function quickly sets up the download and returns immediately. Your program doesn't wait. When the download finishes (which might be milliseconds or minutes later), the background download process invokes thecallbackFunction
you provided, giving it the downloaded data or an error message.
- How it works: You call a function that starts a long task (e.g.,
Highly Flexible and Reusable (Polymorphism & Customization): Passing different callback functions to the same generic function allows that function to perform variations of its task without needing complex
if/else
structures or inheritance hierarchies (though it can complement OOP).Polymorphism (via Callbacks): Implementing varied behavior within a single function by accepting different functions as arguments (callbacks). The specific behavior executed at a certain point is determined by the particular callback function passed by the caller.
- How it works: A function like
process_data(data, operation_callback)
can take your data and then apply anyoperation_callback
you give it. Want to square the data? Passsquare
as the callback. Want to log it? Passlog_data
. Want to encrypt it? Passencrypt
. Theprocess_data
function doesn't need to know what the operation is, only that it's a function it can call with the data. This makes theprocess_data
function highly reusable.
Let's look at a pseudocode example from the source, slightly enhanced to show the power:
function process_message(message, action_callback): // ... do some standard processing ... print("Received message: " + message) // Now, execute the custom action provided by the caller action_callback(message) // ... potentially do more standard processing ... print("Finished processing.") // Define different callback actions function log_message(msg): print("LOG: " + msg) function send_alert(msg): print("ALERT: Urgent! " + msg) // Imagine this sends an email/SMS // Using the same process_message function with different callbacks process_message("System started", log_message) // Logs the message // Output: // Received message: System started // LOG: System started // Finished processing. process_message("Critical error detected", send_alert) // Sends an alert // Output: // Received message: Critical error detected // ALERT: Urgent! Critical error detected // Finished processing.
Here,
process_message
is generic. The specific action taken on the message is decided by the caller when they providelog_message
orsend_alert
as the callback. This is a powerful form of customization and polymorphism without traditional class hierarchies.- How it works: A function like
How the Underground Implements Them: Language Support
Callbacks aren't magic; they rely on specific language features to treat functions as data. The implementation varies, revealing the underlying philosophy of the language.
The Low-Level Secret: Function Pointers: In languages like C, C++, Assembly, Pascal, Modula-2, etc., functions have memory addresses. A function pointer is simply a variable that stores the memory address of a function. You can pass this pointer around, and the code receiving it can "dereference" the pointer to execute the function at that address.
Function Pointer: A variable that stores the memory address of a function. It allows functions to be passed as arguments, stored in data structures, and returned from other functions, effectively treating functions as data.
- Why it's powerful: Direct access to the function's location in memory. Enables seamless integration between code compiled by different compilers or even written in different languages (like C libraries used by other languages via APIs like the Windows API). This is the rawest form of callback.
- Caveats: Type safety can be a concern in C (calling a pointer with the wrong arguments can crash). C++ adds more type safety.
The Modern Flexibility: Function Objects / First-Class Functions: Many dynamic languages (JavaScript, Python, Lua, Perl, PHP), functional languages (Scheme, ML), and newer versions of others (C++, C#, Java, Ruby) treat functions as "first-class citizens." This means functions can be assigned to variables, passed as arguments to other functions, and returned as values from functions, just like numbers or strings.
First-Class Function: A property of a programming language where functions are treated as values that can be passed as arguments, returned from functions, and assigned to variables. This is the foundation for easily using functions as callbacks.
Function Object (Functor): An object that can be called as if it were a function. In languages like C++ or Python, objects can be designed to implement a method (like
operator()
in C++ or__call__
in Python) that allows instances of the object to be invoked like a function. This provides a way to bundle data (the object's state) with the callable behavior, offering more context than a simple function pointer.- Why it's powerful: Much cleaner syntax than function pointers. Seamless integration into the language's data structures. Allows for more expressive code.
The Structured Approach: Delegates: Languages like C# and VB.NET introduce
delegates
. These are type-safe references to methods. A delegate defines a signature (what return type and parameters the method must have), and you can create delegate instances that point to any method matching that signature.Delegate: In languages like C# and VB.NET, a type-safe object that encapsulates a reference to a method. Delegates are used to pass methods as arguments and are fundamental to event handling in these languages.
- Why it's powerful: Provides strong type checking at compile time, preventing errors common with raw function pointers. Supports multicast (a single delegate instance can hold references to multiple methods, and invoking the delegate calls all of them). The foundation of the .NET event system.
The Object-Oriented Pattern: Interfaces: In older object-oriented languages or scenarios where first-class functions weren't available (like Java before version 8), the callback pattern was implemented by defining an
interface
with one or more methods. The function expecting a callback would accept an object that implements this interface. The methods of this object then serve as the callbacks.- Why it's powerful: Leverages the core principles of OOP (polymorphism, encapsulation). Allows callbacks to carry state within the implementing object.
- Caveats: Can be more verbose than function pointers, first-class functions, or delegates due to the need to define a separate interface and often an anonymous inner class or separate class to implement it.
The Anonymous Power: Lambda Expressions (Anonymous Functions): Many modern languages support creating functions "inline" without giving them a formal name. These are lambda expressions. They are frequently used as callbacks because they allow you to define the required custom logic right at the point where you need to pass the callback.
Lambda Expression (Anonymous Function): A function that is not bound to an identifier (a name). Lambda expressions are often used for short, simple callback functions that are defined and passed inline where they are needed.
Capturing Context: Closures: A lambda expression (or a function defined inside another function) in many languages can become a
closure
. This means the function "remembers" and can access variables from the scope where it was created, even if it's executed later in a different scope.Closure: A function that bundles together with references to its surrounding state (the lexical environment). This allows the function to access variables from the scope in which it was defined, even when it is called from a different scope.
- Why it's powerful: Allows callbacks to carry context from where they were created. For example, an event handler created inside a loop can "remember" the specific item being processed in that iteration of the loop.
Cracking the Code: Practical Examples
Let's look at some examples to see callbacks in action. Pay attention to how the function is passed as data and how it's invoked internally.
C: The Foundation (Function Pointers)
#include <stdio.h>
// Define a type for the callback function signature
typedef int (*IntCallback)(); // A pointer to a function that returns int and takes no arguments
// Function that accepts a callback
void print_number(IntCallback get_number) {
// Call the callback function to get the value
int value = get_number();
printf("Value: %d\n", value);
}
// A function to be used as a callback
int get_answer_to_most_important_question() {
return 42;
}
int main() {
// Pass the get_answer... function (its address) as the callback
print_number(get_answer_to_most_important_question);
// Example of a different callback (if we had one matching the signature)
// print_number(get_another_number_source);
return 0;
}
In C, we explicitly declare a function pointer type IntCallback
. print_number
takes an argument of this type. In main
, we pass the name of get_answer_to_most_important_question
. In C, using the function name without parentheses in this context gives you its memory address (a function pointer). print_number
then uses get_number()
to invoke the function pointed to by the get_number
parameter.
- Real-world C Callback Example: Registering a signal handler (
signal(SIGTERM, cleanup_function)
). You tell the OS, "If my program receives a termination signal (SIGTERM
), don't just crash; instead, pause and execute mycleanup_function
(the callback) first."
C#: The Structured Approach (Delegates)
using System;
// Define a delegate type matching the callback signature
public delegate void MessageCallback(string message);
public class Helper
{
// Method that accepts a delegate (callback)
public void ProcessMessage(string msg, MessageCallback callback)
{
Console.WriteLine("Processing message...");
// Call the callback
callback("Callback was: " + msg);
Console.WriteLine("Processing finished.");
}
}
public class Program
{
// A method to be used as a callback (matches MessageCallback signature)
public static void LogMessage(string data)
{
Console.WriteLine(data);
}
public static void Main(string[] args)
{
Helper h = new Helper();
// Pass the LogMessage method as a delegate
h.ProcessMessage("Hello world", LogMessage);
// Using an anonymous method (lambda) as a callback inline
h.ProcessMessage("Another message", delegate(string data) {
Console.WriteLine("Anonymous log: " + data);
});
// Even shorter lambda syntax
h.ProcessMessage("Yet another", data => Console.WriteLine("Lambda log: " + data));
}
}
C# uses delegate
types. ProcessMessage
takes a MessageCallback
delegate. We can instantiate this delegate with a method (LogMessage
) that matches the signature and pass it. C# also allows anonymous methods and lambda expressions which are syntactic sugar for creating delegate instances inline, making simple callbacks very concise.
JavaScript: The Event-Driven Heartbeat (First-Class Functions & Async)
JavaScript is perhaps the language where callbacks are most pervasive, especially in asynchronous contexts. Functions are first-class citizens.
// Blocking Callback Example
function calculate(num1, num2, operate_callback) {
console.log("Starting calculation...");
// Call the callback to perform the operation
let result = operate_callback(num1, num2);
console.log("Result:", result);
console.log("Calculation finished.");
}
// Callback functions
function sum(a, b) { return a + b; }
function multiply(a, b) { return a * b; }
// Use calculate with different callbacks
calculate(5, 3, sum); // Output: 8
calculate(5, 3, multiply); // Output: 15
// Using an anonymous function (lambda) as a callback
calculate(10, 2, function(a, b) { return a / b; }); // Output: 5
// Shorter lambda syntax (arrow function)
calculate(7, 2, (a, b) => a - b); // Output: 5
This JavaScript example shows synchronous callbacks passed to the calculate
function to determine the operation.
JavaScript: Unlocking Asynchronous Power
Here's where JavaScript callbacks truly shine – handling events and async operations:
// Asynchronous Callback Example (Event Handling)
// Imagine this is a browser button element
const myButton = {
// This is a simplified model of how addEventListener works
eventListeners: {},
addEventListener: function(eventName, callback) {
if (!this.eventListeners[eventName]) {
this.eventListeners[eventName] = [];
}
this.eventListeners[eventName].push(callback);
console.log(`Registered callback for event "${eventName}"`);
},
// Simulate the button being clicked
simulateClick: function() {
console.log("Button clicked!");
// When the event happens, call all registered callbacks
if (this.eventListeners['click']) {
this.eventListeners['click'].forEach(callback => {
// Call the callback, potentially with event data
callback(new Event('click'));
});
}
}
};
// Define a callback function for the click event
function handleClick(event) {
console.log("Callback executed: Button was clicked!");
// You could access event properties here like event.target, etc.
}
// Register the callback - This call returns immediately!
myButton.addEventListener('click', handleClick);
console.log("Program continues while waiting for click...");
// Simulate the event happening later
setTimeout(() => { // setTimeout itself uses an async callback!
myButton.simulateClick();
}, 2000); // Simulate click after 2 seconds
console.log("Program is not blocked, can do other things...");
This example demonstrates the core concept of addEventListener
(a pattern used widely in browsers and Node.js). You provide a function (handleClick
) as a callback. The addEventListener
method stores it and returns immediately. The program continues ("Program continues..."
). Only later, when the event (simulateClick
) occurs, is your stored callback function invoked. This is fundamental to how responsive UIs work.
Other common JavaScript async callback uses mentioned in the source:
- Ajax/
XMLHttpRequest
: Initiating a network request and providing a callback (onreadystatechange
or usingfetch().then(...)
) to be executed when the response arrives. setTimeout
/setInterval
: Scheduling a function (the callback) to run after a delay or repeatedly.
Python: Clean and Flexible (First-Class Functions)
Python treats functions as objects, making callbacks straightforward.
# Blocking Callback Example
def calculate(number, operate_callback):
print("Starting calculation...")
# Call the callback
result = operate_callback(number)
print("Result:", result)
print("Calculation finished.")
# A function to be used as a callback
def square(x):
return x * x
# Use calculate with the square callback
calculate(10, square) # Output: 100
# Using an anonymous function (lambda) as a callback
calculate(5, lambda x: x + 10) # Output: 15
Python's syntax for passing functions is clean. calculate
takes operate_callback
, which is just a variable holding a function object. Calling operate_callback(number)
executes the function passed in. Lambdas offer inline simplicity.
More Examples (Briefly):
- Kotlin: Similar structure to C#, often using higher-order functions (functions that take functions) and lambdas.
- Rust: Uses
Fn
,FnMut
,FnOnce
traits to provide type-safe abstractions for different kinds of callable objects (including closures). - Lua: Functions are first-class. Syntax is simple: pass function variables or define anonymous functions inline.
- Julia: Functions are first-class. Syntax is clean, allowing easy passing of named or anonymous functions.
The diverse implementations across languages highlight that the concept of passing executable behavior as data is powerful and adaptable, regardless of the specific syntax.
Mastering the Callback: Beyond the Classroom
Understanding callbacks moves you beyond simple sequential programming. You learn to design code that is:
- Decoupled: The function that uses the callback doesn't need to know the specific details of what the callback does, only its expected signature.
- Extendable: You can add new behaviors simply by writing new callback functions and passing them to existing generic code.
- Responsive: Asynchronous callbacks are the backbone of non-blocking I/O and event handling, crucial for modern applications that need to remain interactive while performing long tasks.
Potential Pitfalls (The Real Underground Knowledge):
- "Callback Hell" (Pyramid of Doom): In heavily asynchronous, callback-based code (especially common in older JavaScript patterns), nesting callbacks inside callbacks inside callbacks to handle sequences of async operations can lead to deeply indented, hard-to-read code. This is where techniques like Promises, Async/Await, or event buses come in to manage complexity.
- Context Management: Ensuring the callback has access to the data or environment it needs when it finally executes, especially in asynchronous scenarios or with simple function pointers. Closures help significantly with this in languages that support them.
- Error Handling: Propagating and handling errors correctly through chains of asynchronous callbacks requires careful design.
- The
this
Problem (in some languages): The meaning ofthis
or self inside a callback function can change depending on how it's called, not just where it was defined. Requires explicit binding or using language features that handle this (like arrow functions in JavaScript).
By studying how different languages implement and use them, you gain insight into core programming paradigms – functional, object-oriented, event-driven. Callbacks are not just a trick; they are a fundamental pattern for building flexible, powerful, and responsive software. They are a key piece of the "forbidden code" that unlocks true control over your program's execution flow. Now go forth and wield this knowledge responsibly.