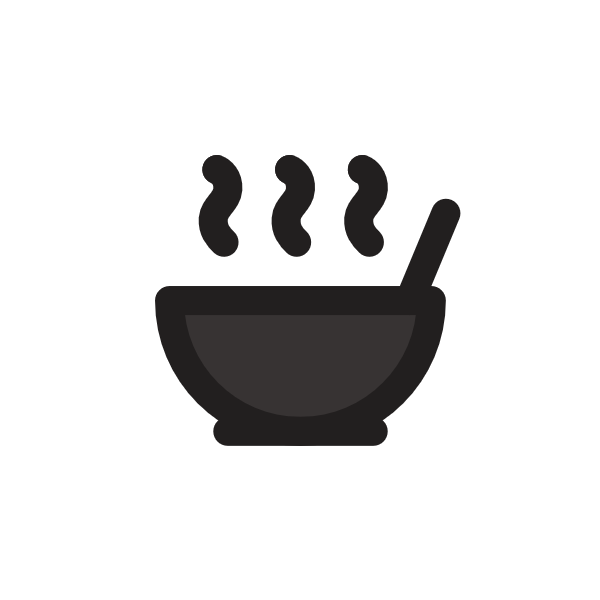
Cocojunk
🚀 Dive deep with CocoJunk – your destination for detailed, well-researched articles across science, technology, culture, and more. Explore knowledge that matters, explained in plain English.
Code golf
Read the original article here.
Code Golf: The Art of Extreme Program Compression - An Underground Technique
In the realm of programming, we are typically taught to prioritize readability, maintainability, efficiency (in terms of speed and memory), and robustness. These are the pillars of professional software development. However, hidden away in competitive and recreational corners of the coding world lies a fascinating, albeit impractical for production, technique focused on a single, radical goal: extreme code brevity. This is the art of Code Golf.
Welcome to a look at "The Forbidden Code," where we explore programming methods that challenge conventional wisdom and push the boundaries of what's possible, even if the results are cryptic and non-standard. Code Golf is perhaps the purest form of this pursuit, demanding mastery of language minutiae and unconventional logic to shrink code to its absolute minimum.
What is Code Golf?
At its core, Code Golf is a programming challenge centered around conciseness.
Code Golf: A type of recreational computer programming competition or activity where participants compete to write the shortest possible source code that correctly solves a given programming problem. The length is typically measured in characters (bytes), but sometimes in keystrokes or other minimal units.
Think of it as programming stripped down to its bare essentials, focusing solely on achieving a functional outcome with the fewest possible symbols. It's an intellectual puzzle that requires deep understanding of a language's syntax, built-in functions, and often, its obscure features or implicit behaviors.
The Golfing Analogy
The name "Code Golf" comes directly from the sport of golf.
Golf Analogy: Just as golfers aim for the lowest possible score (fewest strokes) to complete a course, code golfers strive for the lowest possible character count (fewest "keystrokes" or symbols) to complete a programming challenge.
Unlike most competitive activities where a higher score is better, both conventional golf and code golf reward minimization. This inversion of typical scoring highlights the unique objective: achieving the goal (solving the problem) with the absolute minimal effort in terms of code size.
Historical Roots and Context
While the formal term "Code Golf" gained prominence relatively recently, the underlying concept of striving for minimal code length has a longer history within computing.
One academic parallel exists in the field of algorithmic information theory:
Kolmogorov Complexity: The length of the shortest possible computer program that produces a given output on a fixed universal Turing machine. Named after mathematician Andrey Kolmogorov, this concept from 1963 deals with the inherent minimum size required to generate a specific piece of data, regardless of input.
While related in its focus on minimum representation, Code Golf is often broader than Kolmogorov Complexity. Code Golf problems usually specify an input-output transformation (a function or program) rather than just requiring the generation of a fixed output. The challenge is to find the shortest program that performs this transformation for any valid input.
Informal size optimization challenges were present even in early computing. APL (A Programming Language), known for its concise syntax and powerful array operations using special symbols, was a fertile ground for what could be considered early "golfing" among hackers seeking elegant, compact solutions. The competitive drive for size was noted in official contexts too; a 1962 manual for Denmark's GIER computer explicitly mentioned it as a "time-consuming sport to code with the least possible number of instructions," and notably recommended against it for practical purposes – a sentiment that echoes why it remains an "underground" technique today.
The term "code golf" itself is reported to have first appeared around 1999, gaining traction particularly within the Perl community, which, like APL, lends itself well to concise (and sometimes cryptic) expressions. A notable early example involved golfing a program to perform RSA encryption.
Dedicated Golfing Languages
The pursuit of extreme brevity has led to the creation of programming languages designed specifically for this purpose.
Dedicated Golfing Language: A programming language intentionally designed with features and syntax optimized for writing extremely short code, often sacrificing readability and standard programming conventions for conciseness. These are typically Turing-complete but may have highly specialized or esoteric syntax.
These languages are the specialized clubs in the code golfer's bag, built from the ground up to make common programming tasks expressible in very few characters. Features might include:
- Single-character commands: Mapping complex operations (like mapping a function over a list, sorting, or common I/O) to a single symbol.
- Implicit input/output: Automatically handling standard input and output without explicit commands.
- Stack-based operations: Using a stack implicitly reduces the need for explicit variable names and function arguments.
- Built-in constants and sequences: Providing easy access to common numerical sequences (primes, Fibonacci, etc.) or constants.
- Minimalist syntax: Reducing or eliminating the need for parentheses, semicolons, curly braces, or keywords where possible.
Examples of such languages include:
- GolfScript: An early example, stack-based, inspired by Forth and APL.
- Vyxal: A modern, powerful stack-based language with a large number of built-in functions.
- 05AB1E: Another popular stack-based golfing language.
- Husk: A functional golfing language.
- Pyth: A Python-inspired golfing language.
- CJam: A Java-based golfing language.
- Jelly: A successor to K/APL, known for its concise functional syntax.
- Flogscript, Stuck (also mentioned in the source).
These languages are often considered esoteric due to their highly specialized design, which prioritizes character count over readability and ease of learning. While some languages designed for practical purposes (like APL or Perl) turned out to be suitable for golfing, dedicated golfing languages are built with only golfing in mind. Some challenges even take place on platforms where users can create their own novel golfing language dialects, further pushing the boundaries of conciseness within specific problem constraints (like REBMU, a dialect of REBOL used for "open" questions).
The Techniques of the Code Golfer
So, how do code golfers achieve these astonishingly short programs? While specific techniques vary wildly by language, the general principles revolve around leveraging every possible shortcut:
- Abuse Language Features: Find and exploit lesser-known syntax, implicit type conversions, default behaviors, and built-in functions that happen to perform part of the required task concisely.
- Eliminate Whitespace and Redundancy: Remove all non-essential spaces, newlines, semicolons (if optional), and parentheses.
- Short Variable Names: Use single-character variable names (e.g.,
i
,x
,a
) or avoid variables entirely by chaining operations. - Clever Algorithms: Find mathematical or logical shortcuts that reduce the steps needed, which often translates to fewer characters. Sometimes a slightly less obvious algorithm is much shorter to implement.
- Leverage Standard Libraries/Built-ins: Use powerful built-in functions or library calls that perform complex operations in a single command. Dedicated golfing languages excel here.
- Abuse Errors/Side Effects (Sometimes): In some contexts, a program might crash or behave unexpectedly after producing the required output, and if the competition allows, this might be acceptable. (This is truly "forbidden" territory!).
- Character Encoding Tricks: Sometimes, specific non-ASCII characters in a language's source encoding can represent frequently used tokens or commands, saving bytes.
Consider a simple task like printing the numbers from 1 to 10.
- A standard Python loop might be:
for i in range(1, 11): print(i)
(approx 35 characters) - A golfed Python version could be:
for i in range(11):print(i or 10)
(approx 30 characters, slightly shorter but different logic) - A GolfScript version might be
10,{).p}%;
(approx 10 characters, using stack operations and built-ins). Note: Understanding this requires knowing GolfScript syntax.
The goal isn't to write code anyone can easily read or maintain; it's purely about functional density.
Types of Code Golf Challenges
Code Golf challenges can take various forms:
- Language-Specific: The problem explicitly requires a solution in a particular programming language (e.g., "Golf this in Python," "Shortest Ruby code for X"). This allows problem designers to tailor challenges that leverage specific language features.
- Open Challenges: The problem statement doesn't mandate a language. Participants can use any language. This often leads to solutions in dedicated golfing languages or standard languages known for conciseness (like Perl, Ruby, or increasingly, Python or JavaScript when used with golfing techniques).
- Time-Limited: Some competitions impose time limits on execution or submission, adding another layer of constraint.
The problems themselves can range from simple text manipulation or mathematical sequence generation to complex parsing tasks or graphical output.
Why is This "Underground"? (The Forbidden Perspective)
Given the techniques involved and the resulting code, it's clear why Code Golf is not taught in introductory programming courses or practiced in typical software development teams:
- Readability: Golfed code is notoriously difficult to read and understand. Variable names are minimal, syntax is compressed, and logic is often non-obvious or relies on obscure features.
- Maintainability: Code that can't be easily read is nearly impossible to maintain, debug, or modify later.
- Portability: Golfed code often relies heavily on specific language versions, implementations, or even character encodings.
- Performance/Efficiency: While sometimes a shorter algorithm is faster, often golfing techniques prioritize character count over execution speed or memory usage.
- Collaboration: Golfed code is a nightmare for teams working together.
In professional settings, the costs associated with poor readability, maintainability, and collaboration far outweigh any benefit from saving a few bytes in the source file. Code Golf is an intellectual exercise, a competitive sport, and a way to explore the deep corners of a language's expressiveness – not a methodology for building robust software systems. It's "forbidden" not because it's malicious, but because it actively contradicts the core principles of practical, collaborative software engineering.
Code Golf in Practice and Related Concepts
While not for production code, Code Golf is popular in online communities and competitive programming sites. It serves as a playground for language enthusiasts, a way to learn a language deeply by exploring its limits, and simply a fun challenge.
Code Golf is related to, but distinct from:
Minification (Programming): The process of reducing the size of source code (especially for web assets like JavaScript, CSS, and HTML) by removing whitespace, comments, and shortening variable names, typically without changing the underlying logic or sacrificing standard structure as aggressively as Code Golf. Minification is a practical technique for faster loading times; Code Golf is recreational extreme compression.
Esoteric Programming Languages: Languages designed to experiment with weird ideas, be artistic, or serve as a joke, rather than for practical use. Many dedicated golfing languages overlap with this category due to their unusual syntax and design goals.
Code Poetry: The creation of source code that is intended to be artistic or expressive when read by humans, sometimes focusing on brevity or visual patterns, but with aesthetic goals rather than purely functional or size-based ones.
Code Golf pushes programmers to think outside the box, exploit language features in unintended ways, and appreciate the sheer expressive power available within just a few characters. It's a testament to the flexibility and complexity of programming languages, offering a glimpse into a world where the rules are different, and the smallest solution reigns supreme. While you likely won't use these exact techniques in your day job, understanding the mindset can sometimes illuminate new ways to think about language features and conciseness, even within the confines of readable code. It's a fascinating, forbidden path into the extremes of programming expression.