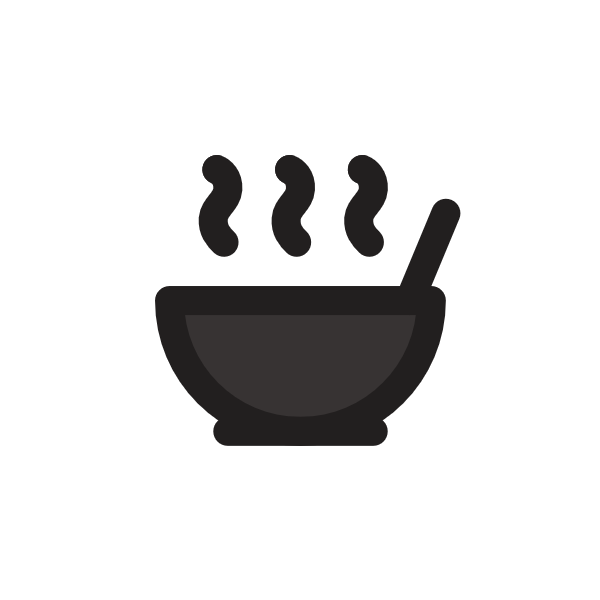
Cocojunk
🚀 Dive deep with CocoJunk – your destination for detailed, well-researched articles across science, technology, culture, and more. Explore knowledge that matters, explained in plain English.
Coroutines
Read the original article here.
The Forbidden Code: Underground Programming Techniques They Won’t Teach You in School
Chapter X: Mastering Non-Linear Control Flow - Unveiling Coroutines
In the hallowed halls of traditional computer science education, you learn the staples: sequential execution, conditional branching, loops, and the venerable subroutine (the function or method you know and love). This forms the bedrock of structured programming, a powerful paradigm that brought order to the chaos of early code.
But beneath this orderly surface lies a realm of techniques offering more granular control, more flexible state management, and alternative approaches to concurrency. These are the techniques that might not fit neatly into an introductory curriculum, often considered too complex or too low-level – The Forbidden Code.
One such powerful, often under-appreciated, mechanism is the Coroutine. While simple functions follow a strict "call and return" lifecycle, coroutines offer a revolutionary concept: the ability to pause execution midway and later resume exactly where they left off. This seemingly small change unlocks incredible possibilities for managing state, building complex systems, and achieving efficient multitasking without the heavy burden of threads.
What Exactly is a Coroutine? Breaking the Function Mold
Let's start by defining this fundamental concept that shatters the traditional subroutine model.
Coroutine: A program component that generalizes subroutines for non-preemptive multitasking, by allowing execution to be suspended and resumed. Coroutines are stackful or stackless. Unlike subroutines, coroutines have multiple entry points for suspending and resuming execution.
Think of a standard function call: you jump into it, it does its work, and it returns once, transferring control back to where it was called, losing all its internal state (local variables etc., unless captured by closures) in the process. A coroutine, however, is like a highly stateful agent. You can jump into it, let it run for a while, tell it to pause (yield control), and then later jump back into it, and it remembers everything – its local variables, its current position, its entire execution context (depending on the type).
This ability to yield control and be resumed later is the core "forbidden" power. It gives the programmer explicit control over the flow of execution in a way that simple functions don't.
Coroutines vs. Subroutines: A Tale of Two Control Flows
Understanding the distinction between coroutines and subroutines (functions/methods) is crucial.
Feature | Subroutine (Function/Method) | Coroutine |
---|---|---|
Entry Point | Single (at the beginning) | Multiple (beginning, and after each yield ) |
Exit Point | Single (return statement, end of function) |
Multiple (yield statements, final return /end) |
State | Local state typically lost upon return | Local state preserved across yield /resume cycles |
Control Flow | Call transfers control, Return transfers back. | Call transfers control, yield transfers control out, resume transfers control back in. |
Lifecycle | Called, runs to completion, returns. | Called, runs, yield s, paused, resumed, yield s again, etc., finally returns. |
In essence, subroutines are about a single, distinct unit of work that completes when called. Coroutines are about ongoing processes that can cooperate, pause, and continue over time.
The Core Mechanisms: Yield and Resume
The magic of coroutines lies in two primary operations: yield
and resume
. (Terminology might vary slightly between languages, e.g., yield
is often the keyword, but resume
is usually an operation performed on the coroutine object).
yield
:- Action: When a coroutine executes a
yield
instruction, it suspends its own execution. - State Preservation: Crucially, before suspending, the coroutine saves its entire current state – this includes its instruction pointer (where it was), and the values of its local variables.
- Control Transfer: Control is transferred back to the entity that resumed or called the coroutine most recently.
- Communication (Optional): A
yield
operation can often return a value back to the resumer.
- Action: When a coroutine executes a
resume
:- Action: When an external entity (usually the caller or a scheduler)
resume
s a coroutine, execution jumps back into the coroutine. - State Restoration: The coroutine restores its previously saved state, including its instruction pointer and local variables.
- Execution Continues: Execution continues immediately after the
yield
instruction that previously suspended it. - Communication (Optional): The
resume
operation can often pass a value into the coroutine, which becomes the result of theyield
expression within the coroutine.
- Action: When an external entity (usually the caller or a scheduler)
# Simple Pseudocode Example
def my_coroutine():
print("Coroutine started")
x = 1
received = yield x # Yield 1, pause, wait for resume with value
print(f"Coroutine resumed, received: {received}")
y = x + received
yield y # Yield y, pause again
print("Coroutine finished")
return "Done" # Final return
# --- External Code ---
# Think of this as the "scheduler" or "caller" managing the coroutine
# "Call" or initialize the coroutine (doesn't run body yet)
coro = my_coroutine()
# Start execution - runs until the first yield
first_yielded_value = next(coro) # In Python, 'next()' starts/resumes coroutines
print(f"Outside: Coroutine yielded {first_yielded_value}")
# Resume the coroutine, sending a value back in
try:
second_yielded_value = coro.send(10) # Send 10 back to the first yield
print(f"Outside: Coroutine yielded again {second_yielded_value}")
# Resume again, potentially finishing
# If the coroutine has no more yields, this will raise StopIteration
coro.send(20) # Send 20 back to the second yield
except StopIteration as e:
final_return_value = e.value
print(f"Outside: Coroutine finished with return value: {final_return_value}")
# Output:
# Coroutine started
# Outside: Coroutine yielded 1
# Coroutine resumed, received: 10
# Outside: Coroutine yielded again 11
# Coroutine finished
# Outside: Coroutine finished with return value: Done
This example illustrates the back-and-forth flow of control and data. The yield
acts as both a pause button and an outgoing message, while resume
(via next
or send
) is the play button and an incoming message channel.
Historical Roots: Coroutines in the Early Days
Coroutines aren't a new invention. They date back to the late 1950s and early 1960s, appearing in languages like Factor and later being formalized by Donald Knuth. They were used in systems programming and simulation languages like Simula before the widespread adoption of threads. In an era of limited memory and processing power, coroutines provided a lightweight way to structure complex interactions and seemingly concurrent processes without the overhead of operating system threads. Their "underground" nature today is partly because threads became the dominant model for concurrency for a time, and structured programming pushed for simpler function call stacks.
Two Flavors of the "Forbidden": Stackful vs. Stackless
Not all coroutine implementations are created equal. A significant distinction lies in how they handle the call stack.
Stackful Coroutines:
- Mechanism: These coroutines essentially have or manage their own execution stack, or they perform complex manipulations of the main program stack.
- Power: Because they save and restore the entire stack, a stackful coroutine can
yield
from anywhere within its execution context, even from inside a function called by the coroutine. - Complexity: Implementing stackful coroutines is significantly more complex, often requiring assembly language or deep operating system interaction to save and restore stack pointers and registers. This complexity is one reason they are less common in high-level language runtimes compared to stackless ones.
- Examples: Languages like Lua, and some library-based implementations in C/C++.
Stackless Coroutines:
- Mechanism: These coroutines do not manage a separate stack. Their state (local variables, current position) is saved and restored explicitly by the compiler or runtime, often by transforming the coroutine code into a state machine.
- Limitation: A stackless coroutine can only
yield
at specific points known to the compiler/runtime, typically directly within the coroutine's top-level function body or within specialawait
points (which are built on coroutines). You usually cannotyield
from a normal, non-coroutine function called by the coroutine, because that function's stack frame isn't part of the coroutine's managed state. - Advantage: Much simpler to implement within existing language runtimes and compilers, often leading to lower overhead. They integrate well with standard function calls.
- Examples: Python generators (
yield
), C#async
/await
, JavaScriptasync
/await
and generators, Kotlin coroutines (often compiled to state machines).
Why this matters: If you're implementing something like cooperative multitasking where tasks might block deep within nested function calls (e.g., waiting for network I/O inside a utility function), you likely need stackful coroutines. If you're building iterators or asynchronous workflows where suspension points are primarily at the top level or marked explicitly (like await
), stackless is usually sufficient and easier to work with.
Use Cases: Why Embrace the "Forbidden"?
Coroutines are powerful tools for specific problems where traditional functions fall short. Their ability to maintain state and pause/resume execution makes them ideal for:
Cooperative Multitasking:
- Concept: Coroutines allow multiple "tasks" to run within a single thread by manually yielding control back to a central scheduler. The scheduler then picks the next task to
resume
. This is cooperative because each task must voluntarily yield; unlike threads, there's no operating system preemption interrupting a task unwillingly. - Advantage: Simplifies reasoning about shared state (no concurrent modification issues between tasks unless a task explicitly yields mid-update), significantly lower overhead than OS threads.
- "Forbidden" Angle: Bypasses the OS scheduler for user-level control over task switching. Requires careful design to ensure no coroutine hogs the CPU.
- Example: Game loops where different game entities (player, enemies, physics simulation) are coroutines that yield after their update step.
- Concept: Coroutines allow multiple "tasks" to run within a single thread by manually yielding control back to a central scheduler. The scheduler then picks the next task to
Implementing Iterators and Generators:
- Concept: Generators are a common application of stackless coroutines. A generator function uses
yield
to produce a sequence of values one by one, pausing its execution after eachyield
and resuming on the next iteration request. - Advantage: Allows creating complex sequences lazily (values are computed only when needed), saving memory compared to generating an entire list upfront. Code looks sequential, even though execution is paused and resumed.
- Example: Reading a very large file line by line without loading the whole file into memory; generating an infinite sequence like prime numbers.
# Python Generator Example (Stackless Coroutine) def simple_generator(): print("Generator starts") yield 1 print("Continues after yielding 1") yield 2 print("Continues after yielding 2") yield 3 print("Generator finishes") gen = simple_generator() print(next(gen)) # Output: Generator starts, 1 print(next(gen)) # Output: Continues after yielding 1, 2 print(next(gen)) # Output: Continues after yielding 2, 3 # print(next(gen)) # Output: Continues after yielding 3, Generator finishes, StopIteration
- Concept: Generators are a common application of stackless coroutines. A generator function uses
State Machines:
- Concept: Coroutines are excellent for implementing systems that transition through different states. Each
yield
point can represent reaching a state or waiting for an event before transitioning. - Advantage: The state (local variables, current position) is naturally managed by the coroutine itself, leading to cleaner code than using explicit state variables and switch/case statements.
- Example: Parsing complex input protocols, managing stages in a workflow, implementing AI behaviors where an agent pauses to wait for something.
- Concept: Coroutines are excellent for implementing systems that transition through different states. Each
Asynchronous Programming (The Modern Renaissance):
- Concept: Modern asynchronous programming models (like C#
async
/await
, JavaScriptasync
/await
, Pythonasyncio
) are often built on stackless coroutines (or similar state machine transformations). Anawait
keyword is essentially ayield
operation that pauses the coroutine until the awaited operation (e.g., network request, file read) completes. When the operation finishes, a scheduler (often an "event loop")resumes
the coroutine. - Advantage: Allows writing asynchronous code that looks synchronous and sequential ("straight-line code"), avoiding "callback hell" and making complex I/O-bound operations much easier to manage and reason about. It enables high concurrency within a single thread, essential for efficient servers and UIs.
- "Forbidden" Angle: Abstract away the complex state management and control flow needed for async operations, but understanding the underlying coroutine concept demystifies how
await
works.
# Python Async Example (Simplified, conceptually based on coroutines) import asyncio async def fetch_data(delay, value): print(f"Fetching data {value} (waiting {delay}s)...") await asyncio.sleep(delay) # <= This is conceptually a yield point print(f"Data {value} fetched.") return value * 2 async def main_async(): print("Starting async operations") # Run tasks concurrently result1 = await fetch_data(2, 5) # <= Await means yield here until fetch_data finishes result2 = await fetch_data(1, 10) # <= Yield here until fetch_data finishes print(f"Received results: {result1}, {result2}") # This runs the 'main_async' coroutine # asyncio.run(main_async()) # Output (approx): # Starting async operations # Fetching data 5 (waiting 2s)... # Fetching data 10 (waiting 1s)... # Data 10 fetched. # Data 5 fetched. # Received results: 10, 20
Notice how
fetch_data(1, 10)
starts whilefetch_data(2, 5)
is still waiting. Theawait
points allow the program to switch between these "tasks" without blocking the single thread.- Concept: Modern asynchronous programming models (like C#
Comparing Coroutines to Other Constructs
- Coroutines vs. Threads: Threads imply OS-managed preemption (the OS can interrupt a thread at any time). Coroutines imply user-managed cooperation (the coroutine yields when it decides to). Threads are heavier (require significant OS resources); coroutines are lighter. Threads are for parallelism (running on multiple cores simultaneously); coroutines are primarily for concurrency (managing multiple tasks on a single core without blocking).
- Coroutines vs. Callbacks: Callbacks inverted control flow (you provide a function to be called later). This can lead to deeply nested "callback hell." Coroutines (especially with async/await) allow you to write asynchronous code with a sequential structure, restoring the natural control flow.
Challenges and Considerations
While powerful, coroutines aren't a silver bullet and come with their own complexities, hinting again at why they might be less prominent in introductory courses:
- Debugging: The non-linear control flow can make debugging more challenging. Stepping through code that jumps in and out of coroutines requires debugger support.
- Cooperative vs. Preemptive: In cooperative multitasking, a single infinite loop or long-running computation within one coroutine can block all other coroutines, as there's no preemption. You must rely on coroutines yielding regularly.
- Stack Management: Especially with stackful coroutines, managing stack growth and switching can be complex and error-prone to implement.
- Integration: While stackless coroutines integrate better, mixing coroutine-based asynchronous code with traditional blocking code still requires care.
Conclusion: Mastering the "Forbidden" Control Flow
Coroutines represent a fundamental departure from the rigid call/return model of subroutines. They offer a powerful, flexible, and efficient way to manage stateful, long-running computations and concurrent tasks within a single thread.
By understanding yield
and resume
, the distinction between stackful and stackless implementations, and their key applications in generators, cooperative multitasking, state machines, and modern asynchronous programming, you gain access to techniques that are essential for building high-performance, responsive, and complex systems.
While they demand a deeper understanding of control flow than simple functions, the power they unlock makes them an indispensable tool in the advanced programmer's arsenal – a truly potent technique from the realm of The Forbidden Code. Don't shy away; learning to wield them effectively will elevate your programming capabilities significantly.
See Also
- "Amazon codewhisperer chat history missing"
- "Amazon codewhisperer keeps freezing mid-response"
- "Amazon codewhisperer keeps logging me out"
- "Amazon codewhisperer not generating code properly"
- "Amazon codewhisperer not loading past responses"
- "Amazon codewhisperer not responding"
- "Amazon codewhisperer not writing full answers"
- "Amazon codewhisperer outputs blank response"
- "Amazon codewhisperer vs amazon codewhisperer comparison"
- "Are ai apps safe"