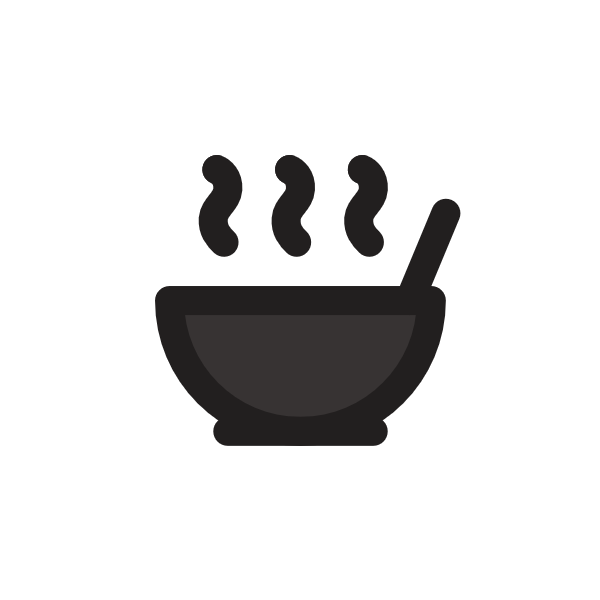
Cocojunk
🚀 Dive deep with CocoJunk – your destination for detailed, well-researched articles across science, technology, culture, and more. Explore knowledge that matters, explained in plain English.
Deadlock
Read the original article here.
The Forbidden Code: Understanding Deadlocks in Concurrent Programming
Welcome to a section of "The Forbidden Code," where we delve into the often-tricky realities of building complex software, focusing on concepts that can cause significant headaches if not properly understood. Today, we tackle Deadlock, a situation that can bring multi-process or multi-threaded applications to a grinding halt, a silent killer in concurrent systems that you absolutely must master to write robust code.
While the term "deadlock" has various meanings outside of computing (like a political impasse or a specific door lock), within the realm of programming, it refers to a very specific and critical problem.
What is a Deadlock (Computer Science)?
Deadlock (Computer Science): A state in which each member of a group of processes is waiting for another member of the group to release a resource, where no member can make any progress because the resources they require are held by other waiting members. Essentially, everyone is waiting for someone else, and no one can move forward.
Imagine a group of friends trying to pass through a narrow hallway, but each one is blocking the path of another, and no one can step aside. That's a physical analogy for a computer science deadlock. In software, the "hallway" is shared system resources (like memory, files, or locks) and the "friends" are processes or threads.
Why is Understanding Deadlock Crucial?
In modern software development, concurrent programming (writing code that can do multiple things seemingly at once) is everywhere. It's essential for:
- Performance: Utilizing multiple CPU cores or distributing work.
- Responsiveness: Keeping an application reactive while performing background tasks (like a UI thread not freezing).
- Handling Multiple Users/Requests: Servers processing numerous client connections simultaneously.
However, concurrency introduces complexity, particularly when multiple processes or threads need to access and modify shared resources. Deadlocks are one of the most insidious bugs in concurrent systems. They don't always happen, making them hard to reproduce and debug. When they do occur, they can cause:
- System Freeze: The affected part of the application or even the entire system becomes unresponsive.
- Resource Starvation: Other parts of the system might be waiting for resources held by the deadlocked processes.
- Data Corruption (Indirectly): While not a direct cause, a frozen state might lead to unexpected program termination or inconsistent state.
Mastering concurrent programming requires understanding how to prevent, detect, and handle deadlocks. It's a mark of a skilled developer moving beyond the basics taught in introductory courses to tackle the real-world challenges of complex systems.
The Four Conditions for Deadlock (Coffman Conditions)
For a deadlock to occur, all four of the following conditions must be present simultaneously in a system:
Mutual Exclusion:
Mutual Exclusion: At least one resource must be held in a non-sharable mode, meaning that only one process at a time can use the resource. If another process requests that resource, it must wait until the resource has been released.
Explanation: This is fundamental. If resources could be used by anyone at any time without restriction (like reading a shared variable without modifying it), deadlocks wouldn't happen over resource access. Deadlocks arise when processes need exclusive control over something, typically achieved using locks, mutexes, or semaphores.
Example: A file opened in write mode. Only one process can write to it at a time. A printer – only one print job at a time.
Hold and Wait:
Hold and Wait: A process must be holding at least one resource and requesting additional resources that are currently being held by other processes.
Explanation: A process doesn't just request all its needed resources at once. It acquires some resources, holds onto them, and then waits for others it still needs. If it had to release all resources before requesting new ones, this condition would be broken.
Example: Process A holds Lock X and requests Lock Y. Process B holds Lock Y and requests Lock X. Both are holding something and waiting for something else held by the other.
No Preemption:
No Preemption: Resources cannot be preempted (forcibly taken away) from a process; they can only be released voluntarily by the process holding them, after that process has completed its task.
Explanation: If the operating system or another process could simply take a resource away from a process that's waiting (without causing issues for the holding process), it could resolve a waiting cycle. But for many resources (like a mutex protecting a critical section of code), this isn't possible or desirable. The resource must be held until the process is finished with it.
Example: You can't suddenly snatch a mutex lock from a thread that holds it without potentially corrupting the data the mutex is protecting.
Circular Wait:
Circular Wait: A set of processes {P₀, P₁, ..., Pₙ} must exist such that P₀ is waiting for a resource held by P₁, P₁ is waiting for a resource held by P₂, ..., Pₙ₋₁ is waiting for a resource held by Pₙ, and Pₙ is waiting for a resource held by P₀. This forms a cycle of waiting.
Explanation: This is the "circle" in the deadlock. The waiting relationships form a closed loop. If P₀ waits for P₁ who waits for P₂ who waits for P₀, you have a simple circular wait with three processes. This condition requires the presence of Hold and Wait and Mutual Exclusion on the resources involved. No preemption ensures the circle remains unbroken.
Example: (Continuing the previous example) Process A waits for Y (held by B), and Process B waits for X (held by A). A → waits for → B → waits for → A. This is a circular wait.
Crucial Point: All four conditions must hold simultaneously for a deadlock to occur. If even one condition is broken, the specific deadlock situation is impossible.
Illustrative Example: The Dining Philosophers Problem
This is a classic computer science problem used to illustrate concurrency issues, including deadlock.
- Scenario: Five philosophers sit around a circular table. In front of each philosopher is a bowl of rice. Between each pair of philosophers is a single chopstick.
- Problem: A philosopher needs both the chopstick to their left and the chopstick to their right to eat. They pick up one chopstick at a time.
- Deadlock Scenario:
- Each of the five philosophers simultaneously picks up the chopstick to their left (Hold and Wait).
- Now, each philosopher is holding one chopstick and waiting for the other chopstick (which is held by the philosopher to their right) (Hold and Wait).
- The chopsticks are resources used under mutual exclusion (only one philosopher can hold a chopstick at a time) (Mutual Exclusion).
- Chopsticks cannot be taken away from a philosopher once picked up (No Preemption).
- Philosopher 1 waits for Philosopher 2's chopstick, P2 waits for P3's, P3 for P4's, P4 for P5's, and P5 waits for P1's chopstick (Circular Wait).
All four conditions are met, resulting in a deadlock. No philosopher can acquire their second chopstick, and thus none can eat or release the chopstick they hold. They are all stuck waiting forever.
Strategies for Handling Deadlocks
Operating systems and application designers employ various strategies to deal with the possibility of deadlocks. These generally fall into three categories:
1. Deadlock Prevention
This strategy aims to design the system or application logic such that at least one of the four Coffman conditions is guaranteed never to occur. This prevents deadlocks by construction.
Break Mutual Exclusion: Generally not possible for resources that inherently require exclusive access (like write access to a file or a critical code section).
Break Hold and Wait:
- Strategy A: Request all resources upfront: A process must request and be granted all the resources it will ever need before it begins execution or at least before acquiring any resource. If any resource is unavailable, it waits, but it doesn't hold anything while waiting.
- Downside: Inefficient resource usage (resources might be held for a long time but only needed later), potential for starvation (a process needing many popular resources might never get them all), requires knowing all future resource needs.
- Strategy B: Release all resources before requesting more: If a process holding resources needs another resource that is unavailable, it must release all the resources it is currently holding and then request the total set again (including the ones it just released).
- Downside: Can cause processes to repeatedly acquire and release resources (thrashing), potentially loss of work if state isn't easily savable upon release.
- Strategy A: Request all resources upfront: A process must request and be granted all the resources it will ever need before it begins execution or at least before acquiring any resource. If any resource is unavailable, it waits, but it doesn't hold anything while waiting.
Break No Preemption:
- If a process requests a resource that's unavailable, it can be preempted (have some or all of its currently held resources taken away). The preempted resources are added to the list of resources for which the process is waiting. The process is restarted only when it can acquire its old and new resources.
- Downside: Only practical for resources whose state can be easily saved and restored (e.g., CPU registers). Difficult or impossible for resources like locks or database transactions without complex rollback mechanisms.
- If a process requests a resource that's unavailable, it can be preempted (have some or all of its currently held resources taken away). The preempted resources are added to the list of resources for which the process is waiting. The process is restarted only when it can acquire its old and new resources.
Break Circular Wait:
- Strategy: Resource Ordering: Impose a total ordering (a numerical or hierarchical ranking) on all resource types. Processes must request resources only in increasing order of enumeration.
- Example: Assign numbers to locks L1, L2, L3... Processes must acquire L1 before L2, L2 before L3, etc. If a process holds L2 and needs L1, it must release L2 first (implicitly breaking Hold and Wait for that resource).
- Downside: Requires assigning an order to all resources, which can be arbitrary and difficult to manage in large systems. Can be inconvenient for developers (e.g., needing Lock B while holding Lock A, but the order says acquire B before A). Does not prevent a process from holding a high-numbered resource indefinitely.
- Strategy: Resource Ordering: Impose a total ordering (a numerical or hierarchical ranking) on all resource types. Processes must request resources only in increasing order of enumeration.
"Forbidden Code" Perspective on Prevention: Prevention strategies, while theoretically sound, can often be complex to implement correctly in a large, evolving system. Forcing a global resource order or requiring upfront resource allocation might reduce concurrency or introduce performance bottlenecks, which developers chasing maximum performance might try to "optimize around," sometimes introducing subtle ways the conditions can still be met. Implementing perfect prevention often requires strict coding guidelines and discipline.
2. Deadlock Avoidance
Deadlock avoidance requires that the system have some prior knowledge about the resources a process will request during its lifetime. The system (typically the operating system) can then dynamically decide whether to grant a resource request or make the process wait, ensuring that the system will never enter an unsafe state – a state from which it is possible for a deadlock to occur.
- Concept: The system analyzes the current resource allocation state and the future resource requests (declared in advance). It grants a request only if there exists at least one sequence in which all processes can complete their tasks without entering a deadlock state.
- Algorithms: The most famous algorithm is the Banker's Algorithm.
Banker's Algorithm: An algorithm used in deadlock avoidance that determines whether a potential allocation of resources to a process is safe or unsafe. It simulates the allocation and checks if all processes can still complete their tasks eventually, given their maximum stated resource needs.
- Requires: Each process must declare the maximum number of resources of each type it might need before it starts executing.
- Downside: Requires significant overhead for checking the safe state upon every resource request. Requires knowing maximum resource needs in advance, which is often impractical in real-world dynamic systems.
"Forbidden Code" Perspective on Avoidance: Avoidance algorithms like the Banker's are elegant in theory but rarely implemented in general-purpose operating systems or application frameworks due to their requirements and computational overhead. Their practicality is limited to systems where resource needs are static and known (e.g., some real-time embedded systems). For typical application development, relying on such system-level avoidance is not feasible. This means developers must handle concurrency issues themselves, often through prevention or detection/recovery.
3. Deadlock Detection and Recovery
This strategy allows the system to enter a deadlocked state, but it includes mechanisms to:
- Detect when a deadlock has occurred.
- Recover from the deadlock.
Detection: The system periodically runs an algorithm to check for cycles in the resource allocation graph (a graphical representation of processes, resources, and their requests/allocations). If a cycle is found involving only processes that are waiting for resources (not actively using them), a deadlock exists.
- Overhead: Running the detection algorithm takes CPU time. The frequency of checking involves a trade-off between timely detection and performance cost.
Recovery: Once a deadlock is detected, the system must break the cycle. Common methods include:
- Process Termination: Abort one or more of the deadlocked processes. The resources held by the terminated process(es) are released, potentially allowing the remaining processes to continue.
- Selection Criteria: Which process to terminate? The one with the least progress, the one holding the most resources, the one that consumed the least CPU time, or simply picking one arbitrarily. Terminating processes can be disruptive (loss of work, system instability).
- Resource Preemption: Forcibly take away resources from one or more deadlocked processes and give them to others. The process that lost its resources might need to restart or roll back its state.
- Downside: Similar issues as breaking No Preemption during prevention – difficult for many resource types.
- Process Termination: Abort one or more of the deadlocked processes. The resources held by the terminated process(es) are released, potentially allowing the remaining processes to continue.
"Forbidden Code" Perspective on Detection & Recovery: This is a pragmatic approach in some systems (like databases, which can rollback transactions involved in a deadlock). For general application development, relying on detection and recovery is often a last resort because recovery (killing processes) is a drastic measure. Developers usually aim for prevention first. However, understanding detection is vital for debugging – using tools that can analyze resource waits (like jstack
for Java threads) is an "underground" technique for diagnosing production deadlocks.
Deadlocks in Specific Contexts
While the four conditions are universal, deadlocks manifest differently depending on the resources involved:
- Threads: Deadlocks are extremely common with threads using locks (mutexes). The classic two-thread, two-lock example (Thread A needs Lock X then Y; Thread B needs Lock Y then X) is a frequent culprit.
- Processes: Processes can deadlock over shared resources like pipes, files, or inter-process communication (IPC) channels.
- Databases: Transactions can deadlock when trying to acquire locks on database rows or tables needed by other transactions that are also waiting for locks. Database systems often use detection and rollback as a recovery mechanism.
- Operating Systems: Processes can deadlock over system resources like memory pages, disk space, or I/O devices.
Practical Tips for Avoiding Deadlocks in Your Code
Since system-level avoidance or detection/recovery is often not available or desirable for application deadlocks, developers primarily rely on prevention within their application logic:
- Consistent Lock Ordering: This is the most practical prevention technique (breaking Circular Wait). Define a strict order for acquiring multiple locks and always acquire them in that order. This is difficult in large codebases but highly effective when manageable.
- Example: If you ever need both
lockA
andlockB
, decide you always acquirelockA
beforelockB
.
- Example: If you ever need both
- Avoid Holding Locks While Waiting: If you hold a lock and need another resource that might take time to become available (like waiting for network data, user input, or another process), release the lock you hold before waiting. Reacquire it after the wait is over. This breaks Hold and Wait.
- Minimize Lock Granularity and Holding Time: Acquire locks for the shortest possible time and protect the smallest necessary sections of code (critical sections). The less time a lock is held, the lower the chance of another process needing it while you wait for something else.
- Use Lock-Free Data Structures: Whenever possible, use concurrent data structures or algorithms that don't rely on traditional locks (e.g., atomic operations, concurrent queues). This removes the possibility of deadlocks related to those specific resources.
- Implement Lock Timeouts: When acquiring a lock, use a method that allows specifying a timeout. If the lock isn't acquired within the timeout, the attempt fails. The process holding resources and failing to acquire the new one can then release its held resources and retry. This helps break Hold and Wait and No Preemption (by simulating preemption via failure and release).
- Thread Pool Management: Be careful with thread pools. If tasks within a thread pool require resources (like other threads from the same pool, or locks held by other tasks in the pool), the pool can deadlock if all threads are waiting for each other.
Conclusion
Deadlocks are not just theoretical computer science curiosities; they are real, system-crashing problems that lurk in concurrent applications. Understanding the four conditions is your key to identifying potential deadlock scenarios in your designs and code. While prevention techniques like consistent lock ordering are often the most viable strategies for application developers, knowing about detection and recovery helps you debug existing issues.
Mastering concurrency and avoiding deadlocks is a sign of true programming skill – the kind they don't always emphasize in introductory courses. It requires careful design, meticulous coding, and a deep understanding of how threads and processes interact with shared resources. Embrace this challenge, and you'll be building significantly more robust and reliable software.