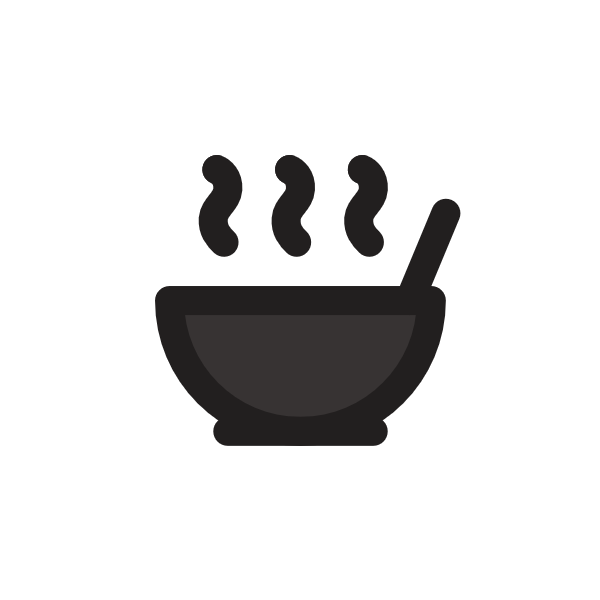
Cocojunk
🚀 Dive deep with CocoJunk – your destination for detailed, well-researched articles across science, technology, culture, and more. Explore knowledge that matters, explained in plain English.
Dynamic dispatch
Read the original article here.
Okay, let's delve into the seemingly simple concept of calling a method, and uncover the "forbidden" truths about what's really happening behind the scenes, focusing on the powerful and sometimes tricky art of Dynamic Dispatch.
While school often teaches you clean, predictable code, the real world (and the most fascinating parts of programming) involve understanding the runtime dance of objects and methods. Dynamic dispatch is one of those dances.
The Runtime Tango: Understanding Dynamic Dispatch
In the pristine world of compiled code, the compiler often knows exactly which piece of code (which function or method implementation) will run when you make a call. You call add(int a, int b)
with two integers, and the compiler points directly to the machine code for integer addition. Simple, right? This is static dispatch.
But what if you're dealing with objects that can take on different forms? What if the actual type of an object isn't known until your program is running? This is where static dispatch breaks down, and where the "forbidden" magic of Dynamic Dispatch comes into play.
Dynamic Dispatch: The process of selecting which specific implementation of a polymorphic operation (like a method or function) will be executed at runtime, rather than at compile time.
Think of it as the program saying, "I know what I want to do (e.g., 'save this record'), but I need to ask the object itself how it wants to do it, because different objects might have different ways of saving."
Why is it "Forbidden"? (Or, Why You Need to Know This)
It's not truly forbidden, of course, but understanding dynamic dispatch goes beyond basic syntax. It's about:
- Understanding Polymorphism's Engine: Dynamic dispatch is the engine that powers polymorphism in object-oriented languages. Without it, you couldn't treat objects of different types uniformly based on a shared interface or base class.
- Performance Implications: Dynamic dispatch involves looking up the correct method at runtime, which inherently has a cost compared to a direct, statically known call. Knowing how it's implemented helps you reason about performance bottlenecks.
- Under-the-Hood Mechanics: Peeking beneath the surface reveals clever data structures (like vtables) and optimization techniques (like caching) that compilers and runtimes use to make dynamic dispatch as fast as possible. This is the kind of knowledge that separates a standard programmer from someone who truly understands the system.
- Designing Flexible Systems: Understanding dynamic dispatch allows you to design systems that are open to extension, where new types can be added easily without modifying existing code that uses the polymorphic interface.
Dynamic vs. Static Dispatch: The Fundamental Divide
The core difference lies in when the decision about which code to run is made:
- Static Dispatch (Compile-Time Binding): The compiler determines the exact function/method implementation to call based on the type information available in the source code before the program runs. This is typically faster because it's a direct jump to a known memory address. It's used for non-virtual functions in C++, overloaded functions, and often for calls to final/sealed methods.
- Dynamic Dispatch (Runtime Binding): The decision is deferred until the program is executing. The system checks the actual, runtime type of the object receiving the call and selects the appropriate implementation defined for that specific type. This is essential for method overriding in object-oriented programming.
Static Dispatch: The process of selecting which implementation of an operation (method or function) to call at compile time, based on the declared type of the variable or expression.
The File/Database Example Revisited
Let's make the example from the source more concrete:
Imagine you're writing a system that processes data. You have different places data can come from or go to: files on disk, entries in a database, maybe even a network stream. All these sources/destinations should support a StoreRecord
operation.
class DataSink:
def StoreRecord(self, record):
raise NotImplementedError("Subclass must implement abstract method")
class FileDataSink(DataSink):
def StoreRecord(self, record):
print(f"Saving record to file: {record}")
# ... actual file writing logic ...
class DatabaseDataSink(DataSink):
def StoreRecord(self, record):
print(f"Inserting record into database: {record}")
# ... actual database insertion logic ...
# In your main program logic:
sink_type = input("Enter sink type (file/db): ") # Type decided at runtime!
if sink_type == "file":
data_sink = FileDataSink()
elif sink_type == "db":
data_sink = DatabaseDataSink()
else:
print("Invalid sink type")
exit()
record_to_save = {"id": 101, "data": "some confidential info"}
# Now, we call StoreRecord. At this point, the program only knows
# 'data_sink' is some kind of DataSink, not its specific type.
# Dynamic dispatch ensures the *correct* StoreRecord implementation is called.
data_sink.StoreRecord(record_to_save)
In this Python example, when data_sink.StoreRecord(record_to_save)
is executed, the Python runtime looks at the actual type of the object currently referenced by data_sink
(which is either FileDataSink
or DatabaseDataSink
) and calls the StoreRecord
method belonging to that specific type. This is dynamic dispatch in action. If it were static dispatch, the compiler would only see data_sink
is a DataSink
and wouldn't know which concrete StoreRecord
to call, which would prevent this kind of flexible polymorphism.
Dynamic Dispatch and Binding: A Subtle Relationship
The Wikipedia article mentions binding. It's important to clarify the relationship:
- Name Binding: Associating a name (like a variable name or a method name) with a specific entity (like a memory location or a piece of code).
- Binding Time: When this association happens.
- Early Binding (Compile-Time Binding): The association is fixed during compilation.
- Late Binding (Dynamic Binding): The association is deferred until runtime.
Late Binding (Dynamic Binding): The process where the linking of a method call to a specific method implementation is performed at runtime.
Here's the key takeaway:
- Dynamic dispatch is the mechanism used when late binding occurs for polymorphic operations. If a call is late-bound, the decision about which implementation to call must happen at runtime, which is exactly what dynamic dispatch does.
- However, you can have languages or situations with dynamic dispatch without full late binding. For instance, in C++, while dynamic dispatch happens for virtual methods, the set of potential methods that could be called is fixed at compile time (you can't add new virtual methods to a class hierarchy or change an object's vtable structure at runtime in standard C++). This is a form of dynamic dispatch operating within an early-bound structure.
So, while late binding implies dynamic dispatch for polymorphic calls, dynamic dispatch doesn't necessarily imply the most flexible form of late binding where method lookups are entirely dynamic and mutable (like in some highly reflective languages).
Single vs. Multiple Dispatch: Whose Type Matters?
Dynamic dispatch can differ based on how many objects' types are considered when choosing the method implementation.
Single Dispatch (Most Common): The method selection is based only on the runtime type of the object on which the method is called (the "receiver" of the message). This is the model used by most popular OOP languages like Java, C#, C++, Python, Ruby, Swift, JavaScript, etc.
- Example (Conceptual):
object.method(arg1, arg2)
. The decision about whichmethod
to run is based only on the type ofobject
, not the types ofarg1
orarg2
. In the File/Database example, the decision fordata_sink.StoreRecord(...)
depends only on the type ofdata_sink
.
- Example (Conceptual):
Multiple Dispatch: The method selection is based on the runtime types of more than one of the arguments involved in the call (often, all arguments). This is less common but found in languages like Common Lisp (CLOS), Dylan, and Julia. This allows for more flexible interactions between objects of different types.
Single Dispatch: A form of dynamic dispatch where the specific method implementation to be called is selected based only on the runtime type of the receiving object (the instance on which the method is invoked).
Multiple Dispatch: A form of dynamic dispatch where the specific method implementation to be called is selected based on the runtime types of multiple arguments involved in the operation, not just the receiver object.
- Example (Conceptual): Imagine a
Collide(ObjectA objA, ObjectB objB)
function. With single dispatch, you might callobjA.CollideWith(objB)
, and the decision depends onobjA
's type. IfobjA
is aAsteroid
andobjB
is aShip
,Asteroid.CollideWith(Ship)
might be called. But what if you want the behavior to depend equally on both? With multiple dispatch, the system would find aCollide
implementation specifically defined for the pair (Asteroid
,Ship
), which might be different fromShip.CollideWith(Asteroid)
or the implementations for (Asteroid
,Asteroid
), etc. This can simplify designing interactions between many object types.
- Example (Conceptual): Imagine a
Multiple dispatch is a powerful tool for problems involving complex interactions, but it adds complexity to the method lookup process.
Peeking Under the Hood: Dynamic Dispatch Mechanisms
How do languages actually perform this runtime lookup? The mechanisms vary, and understanding them is key to grasping the performance characteristics.
C++ Implementation: The VTable Secret
C++ favors speed and compile-time checks. Dynamic dispatch isn't the default; you must explicitly opt-in using the virtual
keyword.
#include <iostream>
#include <vector>
class Shape {
public:
// The 'virtual' keyword enables dynamic dispatch for this method
virtual void Draw() const {
std::cout << "Drawing a generic shape.\n";
}
// Non-virtual method - uses static dispatch
void ReportType() const {
std::cout << "Reporting generic shape type.\n";
}
virtual ~Shape() = default; // Good practice for base classes with virtual methods
};
class Circle : public Shape {
public:
// Overriding the virtual method
void Draw() const override {
std::cout << "Drawing a circle.\n";
}
void ReportType() const { // Hides base class method, static dispatch
std::cout << "Reporting circle type.\n";
}
};
class Square : public Shape {
public:
// Overriding the virtual method
void Draw() const override {
std::cout << "Drawing a square.\n";
}
};
int main() {
// Using base class pointers/references - essential for dynamic dispatch
std::vector<Shape*> shapes;
shapes.push_back(new Circle());
shapes.push_back(new Square());
shapes.push_back(new Shape());
for (const auto& shape : shapes) {
// Dynamic Dispatch: Calls Circle::Draw, Square::Draw, or Shape::Draw
// based on the actual object type pointed to by 'shape'.
shape->Draw();
// Static Dispatch: Always calls Shape::ReportType
// because the type of 'shape' variable is Shape* at compile time.
shape->ReportType();
}
// Clean up allocated memory
for (const auto& shape : shapes) {
delete shape;
}
return 0;
}
Output of the C++ example:
Drawing a circle.
Reporting generic shape type.
Drawing a square.
Reporting generic shape type.
Drawing a generic shape.
Reporting generic shape type.
Notice how Draw()
call dispatches dynamically, but ReportType()
does not.
Virtual Function Table (VTable / VTable): A common, though implementation-specific, data structure used by C++ (and other languages like C# and Java) to implement dynamic dispatch for virtual methods. Each class with virtual methods has a vtable, which is an array of pointers to the implementations of its virtual methods. Each object of that class (or its derived classes) typically stores a hidden pointer (the "vptr") to the appropriate vtable for its actual type.
When you call a virtual method through a base class pointer or reference (shape->Draw()
in the example):
- The system accesses the hidden vptr within the
shape
object. - This vptr points to the vtable corresponding to the actual runtime type of the object (e.g.,
Circle
's vtable). - The system finds the entry for the
Draw
method in that vtable (all classes in the hierarchy agree on the index for a given virtual method). - It follows the function pointer stored at that index in the vtable.
- This leads to the correct
Draw
implementation for the object's actual type (Circle::Draw
,Square::Draw
, orShape::Draw
).
This mechanism is quite efficient – essentially a few pointer dereferences. However, because C++ uses early binding, the structure of the vtable and the methods available through it are fixed at compile time. You can't typically add new virtual methods or change an object's vtable pointer dynamically after compilation.
Go, Rust, Nim: Fat Pointers and Decoupling
These languages use a variation that offers more flexibility than C++'s typical approach, especially when dealing with interfaces or "trait objects."
Instead of the object itself containing the vptr, the reference or pointer to the object carries the necessary dispatch information alongside the data pointer. These are often called "Fat Pointers."
Fat Pointer: A pointer that contains more information than just a memory address. In the context of dynamic dispatch for interfaces/trait objects, a fat pointer typically includes both a pointer to the actual data of the object and a pointer to a "method table" or "vtable" specific to the interface or trait being used.
When you have a variable that refers to an object via an interface (e.g., in Go) or a trait object (in Rust), the variable isn't just a single memory address. It's a pair:
- A pointer to the object's data (
*data
). - A pointer to a vtable (
*vtable
) specific to the interface (Go) or trait object (Rust) being used. This vtable contains pointers to the implementations of the methods defined in that interface/trait, as provided by the concrete object's type.
package main
import "fmt"
// Define an interface (like a contract)
type Speaker interface {
Speak()
}
// Concrete type 1 implementing the interface
type Dog struct{}
func (d Dog) Speak() {
fmt.Println("Woof!")
}
// Concrete type 2 implementing the interface
type Cat struct{}
func (c Cat) Speak() {
fmt.Println("Meow!")
}
func main() {
// Declare variables using the interface type
var animal1 Speaker
var animal2 Speaker
// Assign objects of different concrete types
animal1 = Dog{}
animal2 = Cat{}
// Call the method via the interface.
// This uses dynamic dispatch via fat pointers.
animal1.Speak() // Dispatches to Dog's Speak()
animal2.Speak() // Dispatches to Cat's Speak()
}
In Go's animal1 Speaker
or Rust's dyn Speak
, the variable itself is a fat pointer. When animal1.Speak()
is called, the runtime uses the vtable pointer within the animal1
fat pointer to find the Speak
method implementation for the Dog
type.
Advantages of this approach:
- Decoupling: Libraries or code segments only need to know about the specific interface/trait vtable they require, not the entire concrete type definition. This improves modularity.
- Flexibility: A single object can potentially be referenced by different fat pointers corresponding to different interfaces, each carrying a vtable specific to that interface.
Trade-offs:
- Overhead: Every interface or trait object reference is larger (two pointers) than a simple data pointer, which can add memory overhead, especially if many such references are stored.
- Heap Allocation (Often): To create a trait object (
dyn Trait
) in Rust, the object's data often needs to be on the heap so its size is uniform regardless of the concrete type, allowing the fat pointer to reference it consistently.
Smalltalk & Dynamic Languages: The Lookup and Cache Game
Languages like Smalltalk, Python, Ruby, and JavaScript are often more dynamically typed and highly flexible. Objects can sometimes have their behavior changed at runtime, or even on a per-instance basis (prototype-based languages like JavaScript).
In these languages, method dispatch is fundamentally a runtime lookup process:
- When a message (method call) is sent to an object, the runtime looks at the object's type (or sometimes the instance itself).
- It then searches a data structure (like a dictionary or map associated with the type/object) that maps method names (selectors) to their corresponding code implementations.
- Once the method is found, it's invoked.
- If not found in the object's type, the search might continue up an inheritance chain.
A naive implementation of this lookup could be slow, involving dictionary lookups or traversing inheritance hierarchies on every method call. This is the potential performance cost people often associate with dynamically typed languages.
The "forbidden" optimization technique here is Caching, particularly Inline Caching:
Inline Caching (IC): An optimization technique used in dynamic language runtimes to speed up dynamic dispatch. When a method call site (a specific line of code where a method is called) is executed for the first time, the runtime performs the full method lookup. It then caches the result (the method's address and the receiver's type) directly at the call site. On subsequent calls from that same site, the runtime first checks if the receiver's type matches the cached type. If it does, it directly calls the cached method address, skipping the full lookup process. If it doesn't match (a "cache miss"), it performs a full lookup, updates the cache, and then calls the method.
How it Works: The compiler or JIT (Just-In-Time) compiler essentially rewrites the method call instruction in memory. Initially, it points to a generic lookup routine. After the first call to a certain object type, the lookup routine patches the instruction to directly call the specific method found. It also often inserts a check before the call to quickly verify if the next object has the same type as the one that was cached.
Benefits: If the same method call site repeatedly receives objects of the same few types (which is very common in practice), the cache hits significantly reduce the dispatch overhead, making dynamic dispatch almost as fast as static dispatch after the first call.
Out-of-Line Caching: Another technique uses a separate cache table indexed by the receiver's type and the method name (selector) hash. It's less direct than inline caching but still faster than a full hierarchy walk.
This intelligent caching is a critical piece of "forbidden" runtime magic that makes high-performance dynamic languages possible. It's a classic example of trading initial overhead and complexity for significant average-case performance gains by predicting and optimizing for common runtime patterns.
Instance Behavior & Prototype-Based Languages
Some dynamic languages (like Self, the origin of JavaScript's object model) allow methods to be defined or overridden on individual instances, not just types. This is "instance behavior." Dynamic dispatch in these languages might involve checking the instance first before looking up the type hierarchy.
Prototype-based languages achieve inheritance and behavior sharing by having objects link to a "prototype" object. Method lookups traverse this prototype chain. Dynamic dispatch mechanisms, including caching, are adapted to handle this lookup process efficiently.
Performance Overhead: The Cost of Flexibility
Dynamic dispatch, by definition, involves work done at runtime to determine the target method. This lookup process incurs overhead compared to static dispatch, which is a direct jump instruction.
- C++: Vtable lookup is generally very fast (a few memory accesses). The overhead is minimal per call.
- Go/Rust/Nim: Fat pointer dispatch is also fast (a few pointer dereferences using the vtable carried by the pointer). Overhead is minimal per call.
- Dynamic Languages (Python, Ruby, JS): Naive lookup can be slow. However, with sophisticated caching techniques like Inline Caching, the overhead for frequently called methods on consistently typed objects can be reduced significantly, often to a few instructions, approaching static dispatch speed. The worst-case scenario (cache misses, complex inheritance/prototype chains) still represents a potential bottleneck.
Understanding this overhead is part of mastering the "forbidden code." It informs design decisions – knowing when the cost of dynamic dispatch might be too high (e.g., in tight loops of performance-critical code) and when the flexibility it provides is worth it.
Examples in Code (Revisited)
The Wikipedia article mentions examples but doesn't show them. Let's provide them as a learning tool.
Python Example (Simple):
As shown before, Python's default method call mechanism is dynamic dispatch based on the object's type:
class Dog:
def speak(self):
print("Woof (from Dog)")
class Cat:
def speak(self):
print("Meow (from Cat)")
class Duck:
def speak(self):
print("Quack (from Duck)")
def make_animal_speak(animal):
# Dynamic dispatch happens here!
# The runtime looks at the actual type of 'animal'
# and calls the corresponding speak() method.
animal.speak()
# We create a list of different animal types
animals = [Dog(), Cat(), Duck()]
# We iterate and call the same method 'speak' on each.
# The behavior changes dynamically.
for animal in animals:
make_animal_speak(animal)
# This works even if the types are unrelated by inheritance,
# as long as they have a 'speak' method (Duck Typing).
# Dynamic dispatch is the mechanism that enables this.
C++ Example (Virtual Methods):
Shown earlier, demonstrating the use of virtual
keyword and calling through a base class pointer/reference.
// (See C++ implementation section above for the full code)
// Key part demonstrating dynamic dispatch:
Shape* myShape;
myShape = new Circle();
myShape->Draw(); // Dynamically calls Circle::Draw()
delete myShape;
myShape = new Square();
myShape->Draw(); // Dynamically calls Square::Draw()
delete myShape;
In both examples, the code calling the method (make_animal_speak(animal)
or myShape->Draw()
) doesn't need to know the specific type at compile time. Dynamic dispatch handles routing the call to the correct implementation based on the object's actual runtime type.
Related "Forbidden Arts"
Understanding dynamic dispatch opens the door to understanding related concepts:
- Duck Typing: ("If it walks like a duck and talks like a duck, it's a duck.") In dynamically typed languages, dynamic dispatch enables duck typing. You don't need a formal interface or base class; you just need to call a method, and if the object has that method at runtime, dynamic dispatch finds and calls it.
- Double Dispatch: A specific case of multiple dispatch involving two arguments. Often implemented manually in single-dispatch languages using techniques like the Visitor pattern, which simulate multiple dispatch via multiple single dispatches. This is definitely "advanced" or "forbidden" territory in standard OOP courses.
- Method Overriding: This is the core use case dynamic dispatch is designed for in most OOP languages. A subclass provides its own implementation of a method already defined in its superclass, and dynamic dispatch ensures that when the method is called on a subclass object (referenced via a superclass type), the subclass's version is executed.
- Function Overloading: While overloading allows multiple functions with the same name but different parameter lists, the selection of which overload to call is typically done via static dispatch based on the compile-time types of the arguments. It's a form of static polymorphism, contrasting with the dynamic polymorphism powered by dynamic dispatch and method overriding.
Conclusion: Mastering the Runtime Flow
Dynamic dispatch is not just an academic concept; it's the fundamental mechanism that makes object-oriented polymorphism work in practice. It allows you to write flexible code that can operate on objects based on what they can do (their methods) rather than strictly what they are (their exact type).
While it introduces runtime overhead compared to static dispatch, the clever implementation techniques used in modern languages (like vtables and sophisticated caching) minimize this cost significantly for typical workloads.
By understanding dynamic dispatch, you gain insight into:
- Why your polymorphic code behaves the way it does.
- Where potential performance costs might arise.
- How language runtimes perform impressive feats of optimization under the hood.
This knowledge takes you beyond just writing code that works and into the realm of writing code that is efficient, well-designed, and leverages the full power of your chosen language's runtime environment. It's a crucial piece of the "forbidden code" – the understanding of the system's internals that empowers you to build more sophisticated and robust applications.