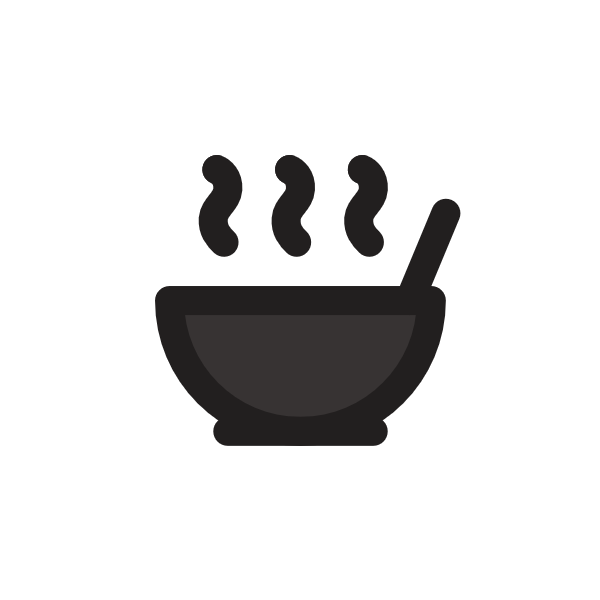
Cocojunk
🚀 Dive deep with CocoJunk – your destination for detailed, well-researched articles across science, technology, culture, and more. Explore knowledge that matters, explained in plain English.
Eval
Read the original article here.
Okay, here is the educational resource on eval
, framed within the context of "The Forbidden Code: Underground Programming Techniques They Won’t Teach You in School".
Executing the Forbidden: A Deep Dive into the eval
Function
In standard programming courses, you learn to write code that the compiler or interpreter reads and executes directly from your source files. This is the foundation. But what if your program needed to generate code on the fly and execute it? What if you wanted to treat data as code? This is where the eval
function, a powerful and often cautioned-against tool, comes into play. It's a technique that can unlock dynamic capabilities but also opens up a pandora's box of security risks and complexity, making it a prime candidate for our exploration of "Forbidden Code".
This resource will dissect eval
, explain its fundamental concept, explore its manifestations across various programming languages, highlight its significant dangers, and touch upon its theoretical underpinnings.
What is eval
? The Core Concept
At its heart, eval
(short for "evaluate") is a function or language construct that takes a piece of data – often a string – and treats it as source code, executing it within the running program's context.
eval
(Evaluate) A function or language construct that parses and executes a string (or other structured representation) as if it were part of the original source code at runtime. It typically evaluates an expression and returns its result.
exec
(Execute) Similar toeval
, but typically used for executing code that consists of multiple statements rather than just a single expression. It may or may not return a value, focusing instead on side effects. Some languages useeval
for both purposes, while others provide distinct functions likeeval
andexec
.
Think of it this way: normally, you write result = 1 + 2;
directly in your code. With eval
, you could potentially have a variable code_string = "1 + 2";
and then use eval(code_string)
to get the result 3
. The code to be executed is determined at runtime, not solely during the initial coding phase.
The input to eval
isn't always a plain string. In some languages, especially those with strong metaprogramming capabilities like Lisp or Python, the input might be a more structured representation of code, such as an Abstract Syntax Tree (AST) or a special "code object" type. This can offer more control and potentially better performance or security than simply parsing a raw string.
The Power and the Peril: Why eval
is "Forbidden"
If eval
is just running code, why isn't it taught more widely or used more freely? The answer lies in the significant risks and complexities it introduces. While it enables dynamic behavior that is difficult or impossible with static code, it sacrifices the safety and predictability of statically defined programs.
The Double-Edged Sword: Security Risks
Using eval
with data that comes from an untrusted source is one of the most common and severe security vulnerabilities in dynamic programming. An attacker could craft a malicious string that, when evaluated by eval
, executes arbitrary code on your system or within your application, potentially taking control, stealing data, or disrupting service.
Consider this simplified, insecure Python example (as mentioned in the source):
# Assume session is a dictionary representing a user's session data
session = {'user': 'guest', 'authenticated': False}
def get_data_from_internet():
# In a real application, this would fetch user input from a web request, API, etc.
# This is the source of untrusted data.
# For demonstration, we'll simulate attacker input:
malicious_input = "session.update(authenticated=True, is_admin=True)"
# benign_input = "1 + 1"
return malicious_input
user_input = get_data_from_internet()
# DANGER! Executing untrusted input directly
result = eval(user_input)
print(f"Session data after eval: {session}")
# Expected output if input was benign: Session data after eval: {'user': 'guest', 'authenticated': False}
# Actual output with malicious input: Session data after eval: {'user': 'guest', 'authenticated': True, 'is_admin': True}
# The attacker has elevated their privileges without proper authentication checks!
Explanation: In this scenario, the program expects to eval
some simple expression or data. However, if an attacker can control the input string (e.g., through a web form, API call, or malicious file), they can inject arbitrary code (session.update(...)
in this case). The eval
function doesn't discriminate; it simply executes whatever valid code it receives. This allows the attacker to manipulate program state (session
dictionary) or even execute commands like os.system('rm -rf /')
.
The
eval
Vulnerability Wheneval
is used with input originating from an external or untrusted source without proper sanitization or restriction, it creates a critical security hole allowing Arbitrary Code Execution. An attacker can craft input that, when evaluated, performs malicious actions within the program's context and permissions.
Mitigation Strategies:
- Avoid
eval
for Untrusted Input: The simplest and often best solution is to never useeval
on input that you do not control and cannot guarantee is safe. If you need to parse data, use dedicated parsers (likejson.parse
for JSON) or safer data formats. - Input Validation and Sanitization: Rigorously check and clean any input string before passing it to
eval
. This is extremely difficult to do perfectly, as you must anticipate all possible malicious code sequences. - Restricted Execution Environment (Sandboxing): Some languages (like Python with its optional
globals
/locals
arguments) allow you to restrict the functions and variables accessible within theeval
'd code. This is a more advanced technique to limit the damage potential, but it's also complex to configure correctly and can still have bypasses.
Because of these significant security implications, using eval
is often discouraged in production code dealing with external data, earning it its "forbidden" reputation in standard programming practices. However, understanding how it works is crucial for both writing secure code (recognizing the vulnerability) and for exploring advanced dynamic techniques.
How eval
is Implemented (Behind the Scenes)
The mechanism behind eval
depends on the language's nature:
- Interpreted Languages: In languages like Python, JavaScript, PHP, etc.,
eval
is typically implemented using the same interpreter that runs the rest of the code. The input string is parsed into an internal representation (like an AST) and then executed by the interpreter engine. - Compiled Languages: For languages that are normally compiled to machine code before execution (like C, C++), implementing
eval
is more complex. It might involve embedding the language's compiler within the runtime environment, allowing it to compile the dynamic code snippet and then execute it. Alternatively, a separate interpreter could be used, but this can lead to code duplication or inconsistencies. Some statically compiled languages, like D, offer related features (mixin
) that operate at compile time rather than runtime.
eval
Across the Landscape: Language Specifics
The behavior and capabilities of eval
vary significantly between programming languages. Let's look at how some popular and less common languages handle this feature.
ECMAScript (JavaScript)
In JavaScript, eval()
is versatile, acting as both an expression evaluator and a statement executor.
// Example as an expression evaluator
let x = 10;
let code_expr = "x + 5";
let result = eval(code_expr); // result will be 15
console.log(result);
// Example as a statement executor
let y = 20;
let code_stmt = "console.log('Value of y is: ' + y); y = 30;";
eval(code_stmt); // Outputs: "Value of y is: 20", then changes y
console.log(y); // Outputs: 30
// A common, but now discouraged, use case: parsing JSON
let json_string = '{"name": "Alice", "age": 30}';
// DANGER: Do NOT use eval for this in production with untrusted data!
// let data = eval("(" + json_string + ")"); // The parens are often needed for expression context
// Use the safer alternative:
let data = JSON.parse(json_string);
console.log(data.name); // Outputs: "Alice"
Context: Historically, eval
was sometimes used in JavaScript frameworks for parsing JSON data received from servers (e.g., in early Ajax). However, this is a prime example of the security vulnerability discussed earlier. Modern browsers and environments provide JSON.parse()
as a dedicated, safe method for this task, which only parses JSON data and does not execute arbitrary code.
ActionScript (Deprecated): The source mentions ActionScript's eval
was quite limited, primarily for retrieving variable/property names rather than arbitrary code execution. ActionScript 3 removed it entirely. This highlights that even when present, eval
isn't always a full-featured code executor.
Lisp
Lisp, being one of the oldest functional programming languages (born in 1958), is intrinsically linked to the concept of eval
. Its very definition and the first interpreter were built around the eval
function. Lisp's code is fundamentally represented as data structures (S-expressions), making the boundary between code and data much blurrier than in most other languages.
Lisp's eval
takes a "form" (a data structure representing code) as its argument and returns the result of evaluating that form.
; Example Lisp code (Common Lisp syntax)
; Define a function
(defun add-numbers (a b) (+ a b))
; Direct evaluation (normal code)
(add-numbers 5 3) ; Evaluates to 8
; Using eval: The argument to eval is a list (a form)
(eval '(add-numbers 5 3)) ; Evaluates the form (add-numbers 5 3), returns 8
; Evaluating a list that is stored in a variable
(let ((my-form '(+ 10 20)))
(eval my-form)) ; Evaluates the form (+ 10 20), returns 30
; Evaluating code from a string - requires parsing the string into a form first
(let ((code-string "(+ 100 200)"))
(let ((parsed-form (read-from-string code-string)))
(eval parsed-form))) ; Parses string to form, then evaluates the form, returns 300
Context: Lisp's design, where code is data, makes eval
a more natural and fundamental operation. The read-eval-print loop
(REPL) is the standard interactive environment for many Lisp dialects, directly reflecting this core process: read
input code (as data), eval
the data (as code), print
the result, and loop
.
Scope: A key consideration in Lisp eval
is the evaluation context (bindings of symbols). Some dialects allow specifying an environment to control which variables and functions are visible to the evaluated code, similar to sandboxing.
Perl
Perl's eval
is another hybrid, handling both expressions and statements. It returns the value of the last evaluated expression.
# Example as an expression evaluator
my $x = 10;
my $code_expr = '$x + 5';
my $result = eval $code_expr; # $result will be 15
print "$result\n";
# Example as a statement executor
my $y = 20;
my $code_stmt = 'print "Value of y is: $y\\n"; $y = 30;';
eval $code_stmt; # Outputs: "Value of y is: 20\n", then changes $y
print "$y\n"; # Outputs: 30
# Note the final semicolon can often be omitted in the eval string
my $code_stmt_no_semi = 'print "Another statement!"';
eval $code_stmt_no_semi; # Works
Context: Perl also uses eval
blocks (syntax: eval { ... };
) for exception handling, which is a completely different usage from evaluating strings at runtime. The code inside eval { ... }
is typically parsed at compile-time.
PHP
PHP's eval()
language construct executes code in a string as if it were part of the surrounding script. It requires complete statements (including semicolons).
<?php
// Example using echo (a statement)
$code_stmt = 'echo "Hello, Eval!";';
eval($code_stmt); // Outputs: "Hello, Eval!"
// Example returning a value (using a return statement within the string)
$x = 10;
$code_return = 'return $x + 5;';
$result = eval($code_return); // $result will be 15
echo "\nResult: " . $result; // Outputs: "Result: 15"
// Note: eval is a language construct, not a regular function
// This means you can't do things like pass it to a higher-order function expecting a callable.
?>
Context: Like other languages, PHP's eval
is frequently a source of vulnerabilities if used with unsanitized user input. It's often seen in older, insecure web applications.
Lua
Lua's loadstring
(or load
in later versions) compiles a string of Lua code into an anonymous function, which can then be executed. This two-step process provides clearer separation between compilation and execution.
-- Example compiling and then evaluating an expression
local code_expr_string = "1 + 2"
local func = loadstring("return " .. code_expr_string) -- Compile string into a function
-- The 'return' is often needed to make it evaluate as an expression with a result
local result = func() -- Execute the compiled function
print(result) -- Outputs: 3
-- Example executing statements
local code_stmt_string = "local x = 10; print(x)"
local func_stmt = loadstring(code_stmt_string) -- Compile statements into a function
func_stmt() -- Execute the compiled function; Outputs: 10
-- Lua 5.2+ uses 'load' which can also take environment as an 'upvalue'
local env = { myvar = 5 }
local func_with_env = load("return myvar * 2", nil, "t", env) -- "t" means text mode
print(func_with_env()) -- Outputs: 10 (accesses myvar from the provided environment)
Context: The loadstring
/load
approach is powerful as it separates the parsing/compilation step (which can catch syntax errors) from the execution step. It also naturally leads to sandboxing possibilities by providing a specific environment (a table in Lua) for the compiled function to run within, limiting its access to the main program's state.
PostScript
PostScript, a stack-based language primarily used for graphics and printing, has operators like exec
. exec
takes an executable object (like a procedure or a string that's been converted to executable) and executes it. To run a string as code, you first convert the string to an executable type.
% Example: converting a string to executable and executing it
% The string "Hello World"
(Hello World)
% The show operator (displays the top of the stack)
show
% Put the string back on the stack
(Hello World)
% Convert the string object (type 'string') to an executable object (type 'executable')
cvx
% Execute the executable object now on the stack
exec
% This sequence pushes "Hello World" string, then converts it to an executable string,
% and then executes that executable string, which contains the PostScript code 'show'.
% The 'show' operator is then executed, displaying "Hello World".
Context: PostScript's exec
fits naturally into its model where code and data are manipulated on a stack. The cvx
operator is key to bridging the gap between a literal string and runnable code. The run
operator serves a similar purpose but executes code directly from a file.
Python
Python distinguishes clearly between evaluating a single expression (eval()
) and executing statements (exec()
). It also provides the compile()
function for more control.
# eval() - evaluates a single expression
x = 10
result = eval("x + 5") # result is 15
print(result)
# eval() with restricted environment (sandboxing attempt)
# __builtins__={} removes access to most built-in functions (like open, exec, eval)
# {'x': x} provides the variable x to the evaluated code
result_restricted = eval("x + 5", {'__builtins__':{}}, {'x': x}) # result_restricted is 15
print(result_restricted)
# Example of restricted environment preventing malicious code (basic)
try:
# Attempt to access a forbidden function
eval("open('secret.txt', 'r')", {'__builtins__':{}}, {})
except NameError as e:
print(f"Caught expected error: {e}") # Access to 'open' is blocked
# exec() - executes statements
y = 20
exec("z = y * 2; print(z)") # Outputs 40, creates variable z in current scope
# print(z) # z is now available in the main scope
# exec() with environment
env = {} # Code runs in this isolated dictionary environment
exec("a = 100; print(a)", env) # Outputs 100
# print(a) # NameError: name 'a' is not defined (a is only in the 'env' dict)
# compile() - converts code string into a code object (useful for repeated eval/exec or sandboxing)
code_string = "print('Hello from compiled code')"
# Compile for execution as a sequence of statements ('exec')
code_object_exec = compile(code_string, '<string>', 'exec')
exec(code_object_exec) # Executes the compiled code object
code_string_expr = "10 * 2"
# Compile for evaluation as a single expression ('eval')
code_object_eval = compile(code_string_expr, '<string>', 'eval')
result_compiled = eval(code_object_eval) # Evaluates the compiled expression object
print(result_compiled) # Outputs: 20
Context: Python's separate eval
and exec
(and compile
) provide granular control. The optional globals
and locals
dictionaries for eval
and exec
are the primary built-in mechanism for creating restricted environments, though proper sandboxing is notoriously difficult to get right and third-party libraries are often recommended for secure scenarios.
D
D, a statically compiled language, does not have a traditional runtime eval
. Instead, it offers the mixin
statement, which evaluates a string at compile time and inserts the resulting code directly into the program's source before compilation proceeds. This is a form of metaprogramming.
import std.stdio;
void main() {
int num = 5;
// Define a string containing code
string code_snippet = "num++;";
// Mixin the string - this code is evaluated *at compile time*
// The compiler sees this line and effectively replaces it with 'num++;'
mixin(code_snippet);
// After compilation and execution, num has been incremented
writeln(num); // Outputs: 6
// The argument to mixin can be any expression resolvable at compile time
mixin("writeln(" ~ "num * 2" ~ ");"); // Outputs: 12 (concatenation evaluated at compile time)
}
Context: mixin
is distinct from runtime eval
. The string's content must be known and valid D code before the program is compiled. It's a powerful tool for generating repetitive code or implementing domain-specific languages integrated tightly with D, but it doesn't allow for executing code based on runtime-determined user input like traditional eval
.
ColdFusion
ColdFusion's evaluate
function is explicitly designed for evaluating string expressions at runtime.
<!--- Example of evaluate --->
<cfset myVariable = "Some Value">
<cfset dynamicVarName = "myVariable">
<!--- Use evaluate to get the value of the variable whose name is in dynamicVarName --->
<cfset variableValue = evaluate(dynamicVarName)>
<cfoutput>#variableValue#</cfoutput> <!--- Outputs: Some Value --->
<!--- More complex example --->
<cfset x = 10>
<cfset code_string = "x * 2 + 3">
<cfset result = evaluate(code_string)>
<cfoutput>Result: #result#</cfoutput> <!--- Outputs: Result: 23 --->
Context: evaluate
in ColdFusion is often used for dynamically accessing variables or properties based on names stored in strings, a form of reflection. While it can evaluate arbitrary expressions, the security implications with untrusted input still apply.
Ruby
Ruby's eval
function is similar to Python and Perl, executing a string as code. A notable feature is the ability to specify a binding (the context of variables and methods) for the evaluation.
# Simple eval
x = 10
result = eval("x + 5")
puts result # Outputs: 15
# eval with a specific binding
def create_binding(y)
binding # Returns a Binding object representing the current scope
end
my_binding = create_binding(20)
# Evaluate code in the scope where y=20 was defined
result_bound = eval("y * 2", my_binding)
puts result_bound # Outputs: 40
# Eval for dynamic class/object extension (advanced, "forbidden" use case)
class MyClass
def hello
"Hello"
end
end
obj = MyClass.new
# Add a new method to the object's singleton class at runtime
eval("def obj.goodbye; 'Goodbye'; end")
puts obj.goodbye # Outputs: Goodbye
# Or add a method to the class itself (affects all instances)
eval("class MyClass; def greet; 'Greetings'; end; end")
puts obj.greet # Outputs: Greetings
Context: Ruby's powerful metaprogramming capabilities make eval
(especially with bindings) useful for advanced techniques like creating dynamic code or modifying classes/objects at runtime. This level of runtime code manipulation is a significant source of flexibility but can make code harder to understand, debug, and secure.
Forth
Forth, a stack-based language known for its extensibility, typically provides words like EVALUATE
and INTERPRET
to process code strings. EVALUATE
usually processes a string using the current interpreter, while INTERPRET
might use a specific interpreter state.
\ Example (Win32Forth syntax)
: MY-DOUBLE ( n -- 2n ) 2 * ; \ Define a word
10 MY-DOUBLE . \ Execute the word: Outputs 20
\ Evaluate a string containing Forth code
S" 10 20 + ." EVALUATE \ Evaluates the string, outputs 30
\ S" ... " pushes the string onto the stack
\ EVALUATE takes the string and executes it
S" MY-DOUBLE 5 + ." EVALUATE \ Evaluates the string, uses the previously defined word, outputs 25
Context: In Forth, extending the language itself is a common pattern. EVALUATE
allows programs to compile and run new definitions or sequences of words provided as strings at runtime, fitting well with the language's interactive and extensible nature.
BASIC Variants
Different BASIC dialects have varied support:
- REALbasic (Xojo): Provides an
RBScript
class, allowing execution of a subset of REALbasic code from a string. It's designed with sandboxing in mind, restricting access to core features unless explicitly allowed via a "context object". This attempts to provide dynamic execution while mitigating someeval
risks. - VBScript: Distinguishes between
Eval
(evaluates an expression, including function calls, potentially with side effects) andExecute
(executes one or more statements). Both can change global state. These were also exposed via the Microsoft Script Control, allowing non-scripting languages to run VBScript or JScript code. - VBA (Visual Basic for Applications): Supports
Eval
only for expressions. It's based on a p-code virtual machine. It can evaluate expressions involving built-in functions, user-defined functions, and objects, but not dynamically named variables (unlike ColdFusion'sevaluate
or VBScript'sExecute
/Eval
). The behavior can subtly differ from VBScript.
Context: The presence of Eval
or Execute
in scripting engines like VBScript and JScript, and their exposure via ActiveX (Microsoft Script Control), was a powerful way to add scripting capabilities to compiled applications. However, this also introduced significant security risks if not carefully managed, contributing to the reputation of dynamic execution features as potentially dangerous.
Smalltalk
Smalltalk, being a highly dynamic and reflective environment where the compiler is often available at runtime, allows for evaluating code strings and even modifying the running system (like adding methods to classes).
"Example: Evaluating a string as code"
Transcript show: (Compiler evaluate: '3 + 4'). "Outputs: 7"
"Example: Dynamically adding a method to a class at runtime"
Object compile: 'myDynamicMethod ^ "Hello from dynamic method"'.
"Now any object can respond to #myDynamicMethod"
'some string' myDynamicMethod. "Returns: 'Hello from dynamic method'"
"Example: Evaluating code within a specific object's context"
| myObject |
myObject := #('apple' 'banana').
(Compiler evaluate: 'self first') in: myObject. "Evaluates 'self first' within the context of myObject"
"Returns: 'apple'"
Context: Smalltalk's philosophy treats the entire system as a set of objects interacting via messages. The compiler itself is an object, allowing code to be compiled and executed dynamically. This capability is deeply integrated into the environment, enabling powerful metaprogramming and live coding practices, representing a language where "code is data" and runtime modification is a first-class citizen, albeit with the inherent complexities.
Tcl
Tcl (Tool Command Language) is designed around the concept that all code is represented as strings, with mechanisms like curly braces {}
used for quoting (preventing evaluation). The eval
command takes a string and executes it as a Tcl script.
# Example: simple eval
set x 10
eval { puts "Value of x is: $x" } ;# Outputs: Value of x is: 10
# Dynamic command creation
set command_name "puts"
set message "Dynamic message"
eval [list $command_name $message] ;# Outputs: Dynamic message
# [list ...] prevents immediate evaluation of variables within the string
Context: Tcl's string-based nature makes eval
a very natural and frequently used command for constructing and executing commands dynamically. While powerful, it carries the same security risks as eval
in other scripting languages if used with untrusted input.
bs (Basic Script)
The bs
(basic script) language from older Unix systems features an eval
function that is both an expression evaluator and a statement executor. It has a unique use for error handling via the ?
operator.
The string argument is evaluated as a
bs
expression. The function is handy for converting numeric strings to numeric internal form. Theeval
can also be used as a crude form of indirection, as in the following (Note that, inbs
,_
(underscore) is the concatenation operator.):abc = 1 var = "abc" eval(var _ " = " _ var _ " + 1")
which increments the variable
xyz
.In addition,
eval
preceded by the interrogation operator,?
, permits the user to controlbs
error conditions. For example:? eval("open(\"XXX\", \"r\")")
returns the value zero if there is no file named "XXX" (instead of halting the user's program).
The following executes a
goto
to the labelL
(if it exists):eval("goto L")
Context: The bs
eval
demonstrates how this concept can be used not just for calculating values or running arbitrary code, but also for dynamic variable access (indirection) and even flow control (goto
). The ? eval(...)
syntax for error suppression is a unique, language-specific feature.
eval
in Command-Line Interpreters
The concept of executing code from a string or arguments isn't limited to programming languages. Command-line shells also have eval
-like capabilities.
Unix Shells (sh
, bash
, zsh
, etc.)
The eval
command in Unix shells concatenates its arguments into a single string, then parses and executes that string as a shell command. This is often used when you need to construct a command string dynamically and then have the shell process it as if it were typed directly.
# Example: dynamically constructing a command string
# Let's say we want to run 'ls -l' in a specific directory determined at runtime
target_dir="/tmp"
command_string="ls -l $target_dir"
# If we just echo it, we see the string
echo $command_string # Outputs: ls -l /tmp
# To execute the string as a command, use eval
eval $command_string # Executes: ls -l /tmp, showing directory contents
# Another common use: handling output that requires a second parsing pass
# e.g., if a command outputs shell aliases or variable assignments
alias myls='ls -l'
# The 'alias' command outputs strings like 'alias myls='ls -l''
# To make the alias effective in the current shell, you need to eval the output
# eval $(alias myls) # Would define the alias 'myls'
Context: Shell eval
is powerful for scripting but requires careful handling of special characters and whitespace, similar to security risks in programming languages. Using it with unsanitized external input (though less common than in web apps) could allow command injection.
PowerShell
PowerShell uses the Invoke-Expression
Cmdlet (iex
alias) to execute a string as a PowerShell command or expression.
# Example as an expression evaluator
$x = 10
$code_expr = "$x + 5"
$result = Invoke-Expression $code_expr # $result is 15
Write-Host $result
# Example as a statement executor
$y = 20
$code_stmt = "Write-Host ('Value of y is: ' + $y); `$y = 30;" # Note escaping $ for assignment in string
Invoke-Expression $code_stmt # Outputs: Value of y is: 20, then changes $y
Write-Host $y # Outputs: 30
Context: Invoke-Expression
provides dynamic command execution in PowerShell. Like eval
in other contexts, using it with untrusted input is a major security vulnerability, enabling attackers to run arbitrary PowerShell commands.
The Theory Behind eval
: Eval-Apply Cycle
In theoretical computer science, particularly in the context of functional programming and lambda calculus, a distinction is often made between two fundamental steps in code execution:
- Eval: The process of taking a representation of code (like a Lisp form or a string) and determining its meaning or value in a given environment. This involves looking up symbols, evaluating sub-expressions, etc.
- Apply: The process of taking a function (which is the result of an eval step) and applying it to its evaluated arguments (which are also the results of eval steps) to compute the final result of the function call.
These two steps are interdependent and form the eval-apply cycle, which is the core operational model for interpreters, especially for languages like Lisp and Scheme. The eval
function needs to call apply
to run the functions it evaluates, and apply
needs to call eval
to determine the value of function arguments.
The famous computer science textbook "Structure and Interpretation of Computer Programs" (SICP) uses the eval-apply
cycle as a fundamental concept to build and explain interpreters.
Potential "Forbidden" Applications (Use with Extreme Caution!)
Despite the warnings, eval
and similar dynamic execution capabilities are not inherently evil. They are powerful tools that enable certain programming patterns that are difficult or impossible otherwise:
- Implementing Interpreters and REPLs:
eval
is fundamental to interactive environments where users type code that is immediately executed. - Metaprogramming: Writing code that writes or modifies other code at runtime (or compile time, as with D's
mixin
). This can be used for code generation, framework development, or advanced optimizations. - Plugin Systems: Allowing users to extend an application's functionality by providing code snippets that the application can load and execute dynamically.
- Dynamic Configuration: Executing configuration files that contain simple expressions or code logic rather than just static data.
- Runtime Code Patching: In highly dynamic environments like Smalltalk or Lisp,
eval
allows for modifying running code without restarting the application, useful for debugging or live updates.
However, every one of these advanced use cases must be implemented with meticulous attention to security and sandboxing if the source of the dynamic code is not entirely trusted. Using eval
without understanding its risks is akin to handling volatile chemicals without safety equipment.
Alternatives to eval
Often, dynamic behavior can be achieved using safer alternatives:
- Configuration Files: Use standard formats like JSON, YAML, XML, or INI for configuration. Parse them with dedicated, safe libraries.
- Domain-Specific Languages (DSLs): Design a mini-language for your specific problem (e.g., a simple calculation language, a rule engine language) and write a dedicated parser and interpreter for only that language. This limits what the dynamic code can do.
- Plugins/Extensions: Use established plugin architectures (like loading shared libraries, specific class interfaces, or message-passing systems) rather than arbitrary code execution.
- Function/Object Registries: Map strings (like command names) to pre-defined, safe functions or object methods rather than evaluating the string as code.
- Template Engines: For dynamic text or code generation, use template languages that separate logic from presentation and limit executable constructs.
Conclusion
The eval
function, and its language-specific equivalents, is a potent tool that allows programs to treat data as executable code. It's a cornerstone of dynamic languages and enables powerful metaprogramming techniques, interactive environments, and flexible architectures.
However, its ability to execute arbitrary code based on runtime input makes it a primary vector for security vulnerabilities, particularly Arbitrary Code Execution attacks. This significant risk is why eval
is often excluded or heavily cautioned against in standard programming curricula, making it a prime example of a powerful but potentially "Forbidden Code" technique.
Understanding eval
is essential not just for wielding its power responsibly in appropriate scenarios, but also for recognizing and mitigating the dangers it poses when misused, especially when handling data from the untrusted outside world. Approach eval
with curiosity, but handle it with extreme caution and respect for the security implications.